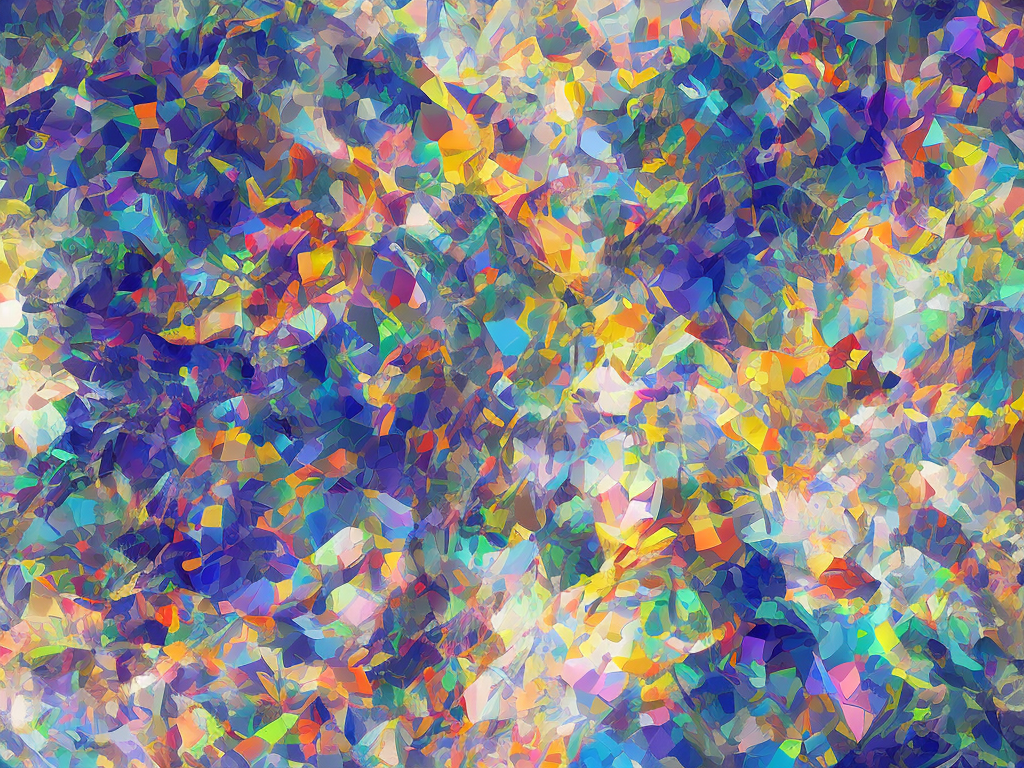
Object-oriented programming (OOP) is a popular programming paradigm that focuses on creating software that is structured around objects, i.e., instances of classes. One of the essential concepts of OOP is the ability to define abstract types. Two of the abstract types that are widely used in OOP are abstract classes and interfaces. While both concepts may seem similar, they have some significant differences. This article discusses the key differences between abstract classes and interfaces.
Definition
An abstract class is a class that cannot be instantiated on its own and is intended to be a base class for other classes to inherit from. In other words, it is a class that is partially implemented and requires its subclasses to provide the implementation for its abstract methods. An abstract class can contain both abstract and non-abstract methods and fields, and it can define constructor(s).
On the other hand, an interface is a pure abstract type that only contains method signatures and constants. It cannot contain any implementation of its methods, and it cannot define constructor(s). An interface is designed to be implemented by classes to provide a common behavior or contract that they all must follow.
Usage
Abstract classes are generally used when creating a class hierarchy. They serve as a blueprint for subclasses to inherit from, providing concrete methods that can be used directly while requiring certain methods to be implemented. Abstract classes are often used when we have a common set of functionalities across different classes, but the implementation of these functionalities differs.
For example, imagine creating a game where there are different types of vehicles. All the vehicles may have common properties like wheels, color, and engine specifications. However, the way they move differs based on the type of vehicle. An abstract class could be created called Vehicle, which can define generic methods such as startEngine(), turnOffEngine(), and getNumberOfWheels(), but the way these methods operate can differ based on the type of vehicle. For instance, a car will start differently than a boat, a boat won't have wheels, etc.
Interfaces are generally used when creating loosely coupled applications or systems. Interfaces define a contract that classes must follow, which provides a level of flexibility to the software design, making it easier to extend without affecting other parts of the system. Interfaces allow for multiple implementations, and thus, they can be used when we have multiple classes with the same behavior.
For example, imagine creating a system that can print documents. We can define an interface called Printable, which will define the method print(). Now, we can create two independent classes, a Printer and a PDFCreator, that will implement this interface. This allows the client code to interact with these classes through their common interface, Printable, without needing to know the details of each class's implementation.
Inheritance
Abstract classes support inheritance, and they are designed to be inherited. Subclasses extend the abstract class and use it as a base class, inheriting both its defined and implemented methods. Abstract classes can also contain non-abstract methods that can be inherited directly.
Interfaces, on the other hand, use implementation to provide behavior to the implementing class. If a class implements an interface, it must provide an implementation for all the methods specified in the interface. Interfaces do not support inheritance in the traditional sense, but they can be extended through other interfaces that inherit them. A class can implement multiple interfaces, but it can only inherit from one superclass.
State
Abstract classes can contain state in the form of fields, which can be inherited and used by the subclasses. These fields are often used to define common properties across the class hierarchy. Subclasses can also override these fields if necessary.
Interfaces cannot contain any fields, so they cannot define state. They are solely for defining behavior and contracts.
Access Modifiers
Access modifiers are used to determine the scope of a class, its members, and its methods. Abstract classes can have any access modifiers, public, protected, private, or default, depending on the purpose of the class.
Interfaces, on the other hand, are always public. All the methods and constants defined in an interface are implicitly public and cannot be changed.
Method Implementation
Abstract classes can contain both abstract and non-abstract methods. Abstract methods must be implemented by the subclasses, while non-abstract methods can be inherited by the subclass or overloaded if required.
Interfaces can only contain abstract methods, which must be implemented by the classes that implement the interface. This ensures that all classes that implement the interface follow a common behavior.
Conclusion
In conclusion, while abstract classes and interfaces share similarities, they are used for different purposes in OOP. Abstract classes are used in class hierarchies as a superclass that provides partial implementation while requiring subclasses to implement the rest. Interfaces, on the other hand, define a contract that classes must follow to provide a common behavior. Interfaces are used to create loosely coupled applications and to ensure that classes follow a common behavior without knowing each class's implementation. Knowing these key differences is fundamental to creating effective object-oriented designs.