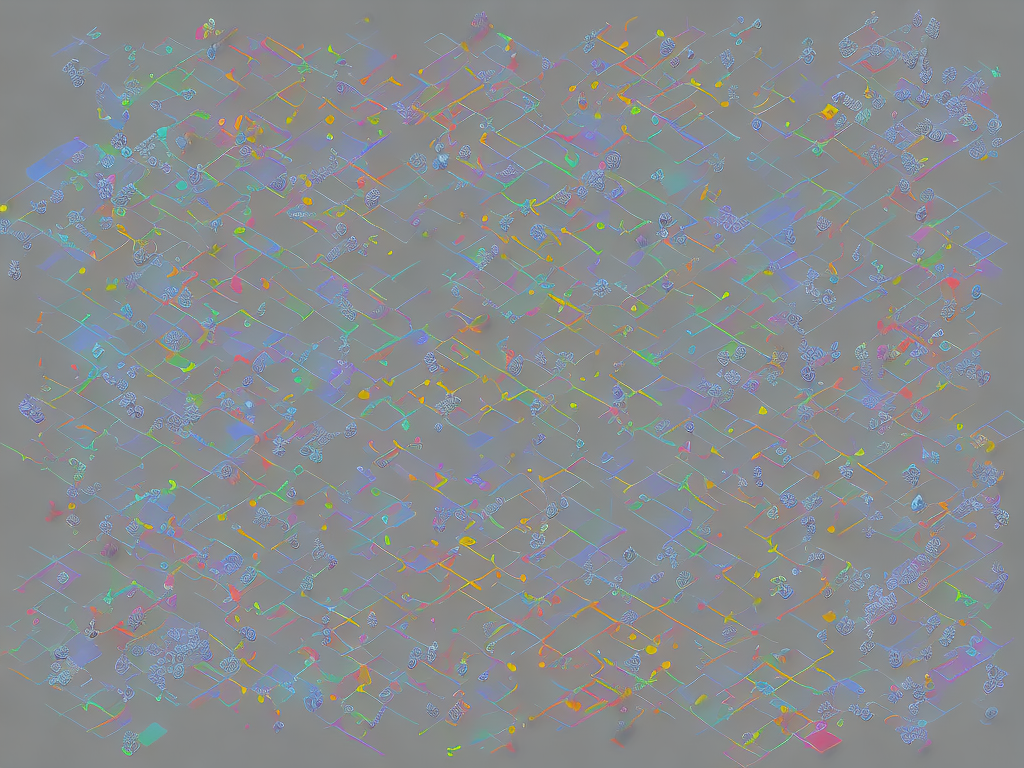
Arrays and linked lists are two fundamental data structures used in computer programming. Arrays and linked lists both offer developers the ability to store data, but there are significant differences between the two. In this article, we will discuss the differences between arrays and linked lists to help you understand which data structure is appropriate for particular scenarios.
Arrays:
An array is a fixed-size data structure that stores a group of elements of the same type. Each element in an array is identified with an index, starting from zero. Typically, developers use arrays when they know the size of the data they want to store in advance. Arrays have some essential characteristics that programmers must understand:
1. Memory allocation: In an array, all elements of the array are allocated in contiguous memory locations. This means that if the size of an array is n, then n contiguous memory locations are allocated at once.
2. Access: Arrays can be accessed using the square bracket notation, which means that the index of an element in an array is used to access its value. The time to access the element is constant time O(1).
3. Insertion and Deletion: Insertion and deletion operations on arrays are slow since all elements must be shifted to make room for the new element or to fill in a gap after deleting an item.
4. Fixed size: Arrays have a fixed size, which is set during initialization, and cannot be changed.
Linked list:
A linked list is an ordered collection of elements, where each element is connected by a link to its successor. Unlike arrays, linked lists are dynamic data structures that allow for efficient insertion and deletion of nodes. Linked lists have several characteristics that make them ideal for certain scenarios:
1. Memory allocation: Linked lists nodes are not stored in contiguous memory locations like arrays. Each node stores two values: the value of the element and a pointer that points to the next node in the list.
2. Access: Accessing an element in a linked list requires traversing the linked list from its head to the desired node. The time complexity for accessing an element is O(n), where n is the number of elements in the list.
3. Insertion and Deletion: Since linked list nodes are not stored in contiguous memory locations, inserting and deleting nodes in a linked list is faster than in an array. Removing or adding a node requires modifying the links of the neighboring nodes keeping the order.
4. Dynamic size: Linked lists have a dynamic size, making them ideal for growing data sets as they can grow or shrink as required.
Key Differences:
1. Memory allocation: As mentioned earlier, Arrays are stored in contiguous memory locations, whereas linked lists's nodes are not stored in contiguous memory locations.
2. Speed: Arrays are faster than linked lists when accessing an element, but linked lists are faster when inserting or deleting an element.
3. Size: Arrays have a fixed size, whereas linked lists' size can be changed during runtime.
4. Memory usage: Arrays have a higher memory usage than linked lists since they need to allocate contiguous memory upfront.
When Should You Use Arrays?
Arrays are a good choice when the size of the dataset to be stored is known in advance, and fast random access is required. Arrays are good for mathematical operations since they offer fast access to their elements. Also, arrays are optimal for searching, sorting, and iterating over all elements in the array.
When Should You Use Linked Lists?
Linked lists are recommended when the size of the dataset is not known in advance and size can grow or shrink dynamically. They're useful when we're not sure exactly what the input size may be. Besides, linked lists are great when insertion/deletion operations need to be performed quickly. They are also used in graph algorithms or for implementing trees and graphs.
Conclusion:
Arrays and linked lists are two essential data structures that every programmer should understand. When choosing between arrays and linked lists, it is essential to consider your project's requirements. Arrays are faster when accessing elements and are optimal for mathematical operations, whereas linked lists are better when inserting or deleting elements. The good news is that, by understanding the differences mentioned in this article, you can now make informed decisions about which data structure to use for a particular situation.