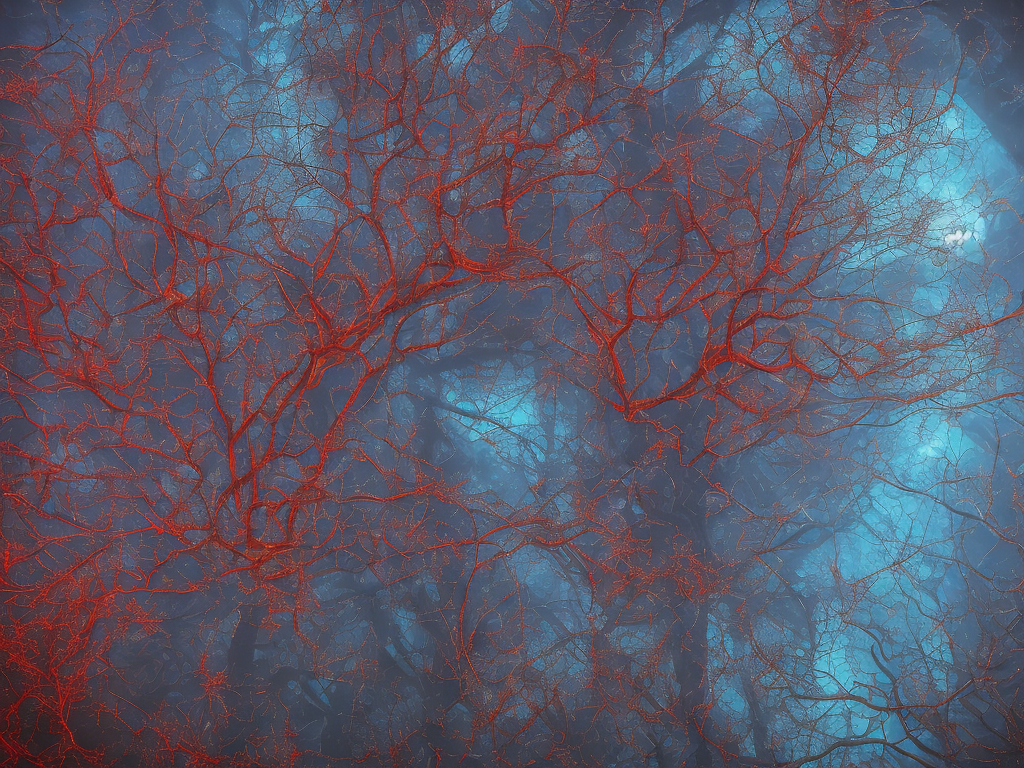
When it comes to graph traversal algorithms, the two most popular ones are breadth-first search (BFS) and depth-first search (DFS). Both algorithms visit every vertex in a graph, but they do so in different ways. In this article, we’ll explore the main differences between BFS and DFS.
BFS
BFS is a graph traversal algorithm that visits all the vertices of a graph in a breadth-first manner. It starts at the root node and explores all the neighboring vertices first before moving to the next level of vertices. BFS uses a queue data structure to keep track of the vertices that are waiting to be visited.
In BFS, we start from the source vertex and visit all the vertices that are directly connected to it. Then, we move on to the next level of vertices, i.e., the vertices that are connected to the vertices of the first level. We continue this process until we have visited all the vertices in the graph. The time complexity of BFS is O(V + E), where V is the number of vertices and E is the number of edges in the graph.
The main advantage of BFS is that it finds the shortest path between the source vertex and all the other vertices in an unweighted graph. This is because BFS visits all the neighboring vertices of a vertex before moving on to the next level. So, if we want to find the shortest path between two vertices in an unweighted graph, BFS is the best algorithm to use.
DFS
DFS is a graph traversal algorithm that traverses a graph in a depth-first manner. It starts at the root node and explores as far as possible along each branch before backtracking. DFS uses a stack data structure to keep track of the vertices that are waiting to be visited.
In DFS, we start from the source vertex and visit one of its neighbors. We then visit the neighbor of the neighbor, and so on until we reach a dead-end. At this point, we backtrack to the previous vertex and explore the other paths that we haven’t visited yet. We continue this process until we have visited all the vertices in the graph. The time complexity of DFS is also O(V + E), where V is the number of vertices and E is the number of edges in the graph.
The main advantage of DFS is that it can be used to solve problems that involve finding cycles in a graph or paths between two vertices in a weighted graph. DFS is also useful to detect strongly connected components in a directed graph.
Differences between BFS and DFS
The main difference between BFS and DFS is the order in which they visit the vertices. BFS visits all the vertices of a graph in a breadth-first manner, while DFS visits them in a depth-first manner.
BFS uses a queue data structure to keep track of the vertices that are waiting to be visited, while DFS uses a stack data structure. In BFS, we visit all the neighboring vertices of a vertex before moving on to the next level, while in DFS, we visit one of the neighbors and explore as far as possible along the branch before backtracking.
The time complexity of BFS and DFS is the same, O(V + E), but the space complexity of BFS is greater than that of DFS. This is because BFS needs to keep track of all the vertices that are waiting to be visited, while DFS only needs to keep track of the vertices on the current path.
BFS is best suited for finding the shortest path between two vertices in an unweighted graph, while DFS is best suited for finding cycles in a graph or paths between two vertices in a weighted graph.
Conclusion
In conclusion, both BFS and DFS are important graph traversal algorithms that are widely used in computer science. They have different strengths and weaknesses, and choosing the right algorithm for a particular problem depends on the characteristics of the graph and the problem itself. In general, if we need to find the shortest path between two vertices in an unweighted graph, BFS is the best algorithm to use. If we need to find paths between two vertices in a weighted graph or detect cycles in a graph, DFS is the best algorithm to use.