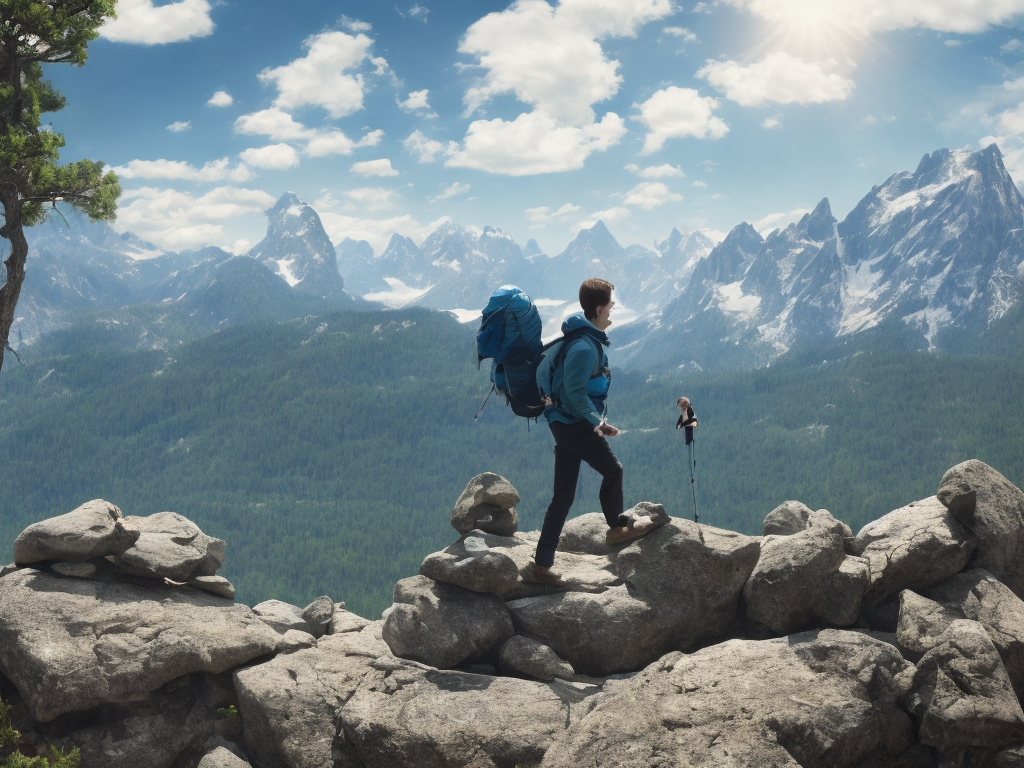
When programming, there are times when you need to change the flow of execution of a loop or a block of code. Two of the most commonly used keywords for such purposes are ‘break’ and ‘continue’. While both of them are used for manipulation of such conditions, they have two distinct functions.
Break:
The keyword ‘break’ is used to exit a loop completely. It is commonly employed in for, while, and do-while loops. The break keyword enables the program to cease ongoing execution of a loop which usually happens when a specific condition is met. The break statement works by immediately terminating the innermost loop in which it is executed, along with any accompanying statements within that loop. The loop, in this case, may be a for loop, do-while loop, or while loop.
The following code gives an example of how break statements can be used in a for loop in Python:
for i in range(10):
print(i)
if i == 5:
break
Output:
0
1
2
3
4
5
Explanation:
The code above generates a loop that runs ten times, printing the numbers 0 to 9. However, the `if` statement that follows the print statement in the loop tells the program to break the loop as soon as it reaches the number `5`. Therefore, the output above shows the program ends at 5.
Other programming languages have a similar implementation of break statements. For example, in Java, the code above would be:
for (int i=0; i<10; i++) {
System.out.println(i);
if (i == 5) {
break;
}
}
Continue:
The ‘continue’ keyword is used to skip a particular iteration of a loop and move on to the next iteration. The continue statement, unlike the break statement, does not exit the loop. Instead, it jumps back to the start of the loop, incrementing the control variable and continuing with the rest of the code.
Let's examine this example in Python:
for i in range(5):
if i == 3:
continue
print(i)
Output:
0
1
2
4
Explanation:
The above program generates a loop that runs ten times, printing the numbers 0 to 4. However, the `if` statement within the loop tells the program to continue with the next iteration as soon as it gets to 3. This causes the program to skip 3 in the output above.
Java has the similar implementation of the continue keyword as well. Here's an example to illustrate this:
for (int i=0; i<5; i++) {
if (i == 3) {
continue;
}
System.out.println(i);
}
Output:
0
1
2
4
Use Cases:
The break and continue statements have several applications in programming. They boost the performance of loops by enabling the programmer to control the way loops run.
When to use break:
The ‘break’ statement is used when you need to exit out of a loop based on a specific condition. It is also beneficial when breaking out of nested loops. The example below shows how the function of the break keyword works in nested loops:
for i in range(2):
for j in range(2):
print(i, j)
if (i == j):
break
Output:
0 0
1 0
1 1
Explanation:
Here, the code generates a nested loop that prints the values 0 to 1 along one axis twice. Nonetheless, the inner loop has a condition that specifies that it should break if the loop's control variable `i` is equal to `j`. Effectively, this leads to 'breaking' out the outlier nested loop immediately the condition is met. The output above shows that the loop breaks at `1 1`.
When to use continue:
The ‘continue’ statement, on the other hand, is used to skip to the next iteration of a loop based on specific conditions. It is best used with performance issues when you need to avoid unnecessary executions of code.
An example of a use case is shown below:
for i in range(5):
if i == 3:
continue
print(i)
Output:
0 1 2 4
Explanation:
In the example above, the loop prints values from 0 to 4. However, whenever the value of the control variable is 3, the `continue` statements skip that iteration, therefore ensuring that '3' is not among the printed values.
Conclusion:
In summary, both 'break' and 'continue' statements are significant in programming. They are two powerful tools that help programmers modify the pattern of loop execution. The difference between them, however, is that 'break' is used to end a loop, while 'continue' is used to skip certain iterations within a loop. Understanding and using these statements effectively will go a long way in optimizing your code and enhancing your software's functionality.