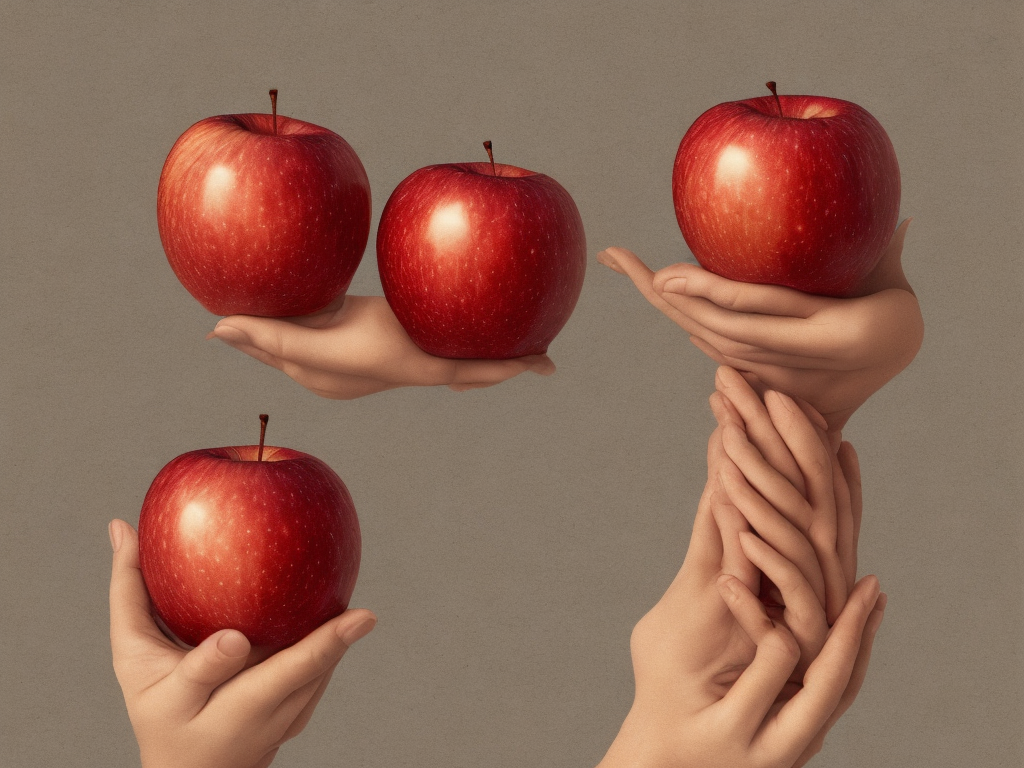
In computer programming, functions are an essential tool for organizing code, optimizing performance, and making it easier to solve complex problems. However, the way that functions work is not always straightforward, especially when it comes to how they handle the input (parameters) and output (return value) of data.
Two common methods for passing data to functions are called call by value and call by reference. While they may seem similar at first glance, there are significant differences between the two that can affect a program's behavior and efficiency. In this article, we will explore the difference between call by value and call by reference.
Call By Value
Call by value is a method of passing data to a function by making a copy of its value and passing that copy to the function. This means that the function receives its own independent copy of the data, which it can modify without affecting the original data outside the function. After the function completes its task, it returns a value, and the original data remains unchanged.
Consider the following example:
```
void increment(int num){
num++;
}
int main(){
int x = 2;
increment(x);
cout << "x = " << x << endl;
}
```
In this example, the function increment takes an integer parameter num and increments it by one. However, since num is passed by value, the variable x remains unchanged after the function is called. The output of the program would be:
```
x = 2
```
This is because the value of x is copied to the function increment, and the changes made to num are not reflected in x.
One advantage of call by value is that it is safer because the function cannot accidentally modify the original data outside its scope. This can be useful when working with sensitive data or when you want to ensure that the original data remains unchanged. Additionally, since the function receives its own copy of the data, it can make changes without affecting the original data, making it easier to reason about code and avoid unexpected results.
The downside of call by value is that it can be slower and less memory-efficient, especially in cases where you need to pass large or complex data structures. This is because copying large amounts of data can be time-consuming and use up a lot of memory. Additionally, if the function needs to modify the original data, it would need to return a new copy of the data, which can also be time-consuming and inefficient.
Call By Reference
Call by reference is a method of passing data to a function by passing a reference to the original data instead of making a copy. This means that the function can directly access and modify the original data, and any changes made to it will be reflected outside the function. Additionally, the function does not return a value in this case; instead, it directly modifies the original data.
Consider the following example:
```
void increment(int& num){
num++;
}
int main(){
int x = 2;
increment(x);
cout << "x = " << x << endl;
}
```
In this example, the function increment takes an integer reference parameter num instead of a copy, which means that any changes made to num will be reflected in x outside the function. When we call the function increment with x as a parameter, it increments x by one, and the output of the program would be:
```
x = 3
```
One advantage of call by reference is that it can be faster and more memory-efficient, especially when working with large or complex data structures since there is no need to make a copy of the data. Additionally, since the function can modify the original data directly, it can reduce code duplication and make functions more reusable.
However, call by reference can also be more error-prone because the function can modify the original data, sometimes resulting in unexpected behavior. If the original data is modified in a way that was not intended or expected, it may be hard to find and fix the issue, resulting in bugs or crashes.
Conclusion
In summary, the main difference between call by value and call by reference is how data is passed to a function. Call by value makes a copy of the data, while call by reference passes a reference to the original data. While call by value can be safer, call by reference can be more efficient and make code more reusable. It is important to carefully consider which method to use based on the specific needs of your program and the type of data you are working with. By understanding these differences, you can write more efficient, effective, and bug-free code.