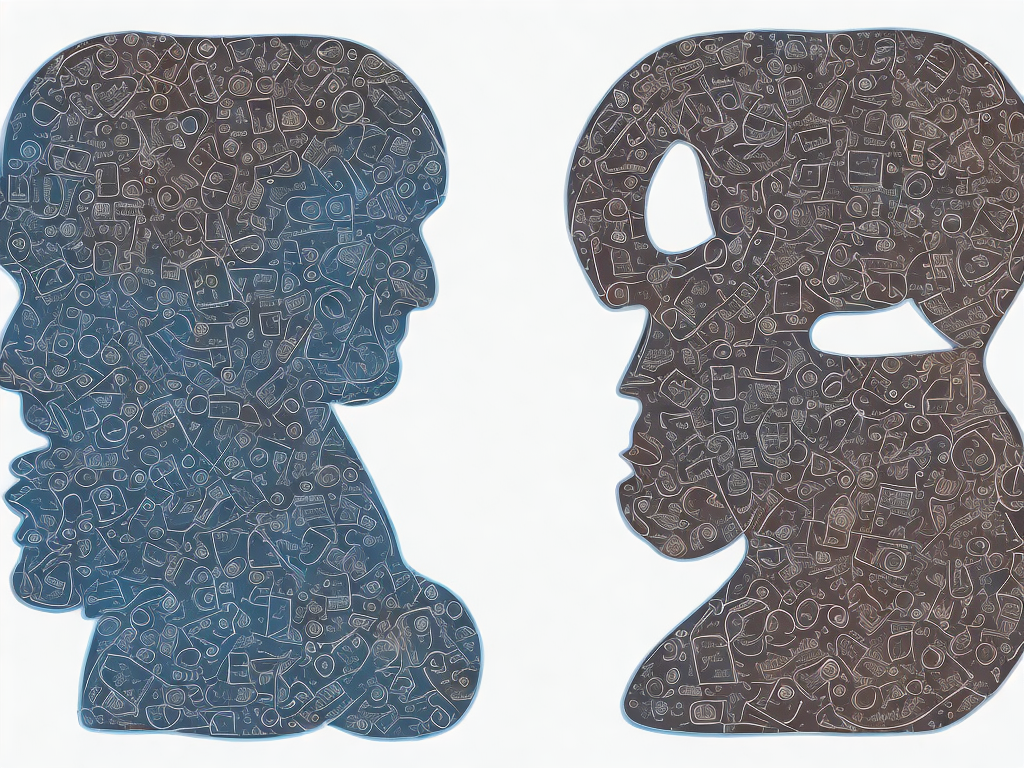
Computers understand binary code, which is a collection of ones and zeroes. However, programming in binary code is not practical. Therefore, programming languages have been developed to help programmers create code that is more human-readable and easier to maintain.
Programming languages such as C#, Java, and Python are known as high-level languages, as they are easier for humans to read and write. However, computers do not understand high-level languages. Therefore, they need to be translated into machine code, which is executed by the computer. This process of translation is done by either a compiler or an interpreter.
Both compilers and interpreters are software tools that programmers use to translate high-level programming languages into machine code. While they achieve this same goal, they do so in different ways, and there are significant differences between compilers and interpreters.
What is a Compiler?
A compiler is a software program that translates source code written in a high-level programming language into machine code. It analyzes the entire source code at once and generates an executable program. The program can then be run multiple times without needing to be re-translated, as the translation process is already completed.
The process of compiling involves four stages:
1. Lexical Analysis - The source code is broken down into a sequence of tokens that define the basic structure of the program.
2. Syntax Analysis - The tokens are analyzed to ensure they are in the correct order and structure.
3. Semantic Analysis - Checks are made to ensure the code is free of errors and that the meaning of the code is correct.
4. Code Generation - Translates the source code into machine code.
Compilers are favored by large applications that are required to execute fast. They remove the need for additional libraries as the executable is self-contained. These applications are also harder to reverse engineer, as the source code is not present in the final product.
What is an Interpreter?
An interpreter is software that reads the source code line-by-line and executes it as it interprets it. Unlike compilers, interpreters do not create an executable file. Instead, they interpret and execute the program as it is being read.
The interpretation process involves the following:
1. Tokenizing the source code
2. Parsing the tokenized code
3. Interpreting the code to execute it
Interpreted languages, such as Python and JavaScript, are popular for their ease of use and availability of libraries. Maintaining an interpreted program is simpler, as the source code is readily available. Bugs and errors can be identified and fixed, resulting in clean code for the user.
Differences Between Compiler and Interpreter
1. Execution time
- Compiled programs run faster as compared to interpreted ones as they are pre-compiled into machine code.
2. Memory usage
- Compiled programs use less memory as they do not need to maintain additional data structures and code during runtime.
3. Debugging
- Debugging an interpreted program is easier as the source code is readily available. Changes can be made and executed immediately without the need for recompilation.
4. Portability
- Interpreted programs are more portable as they do not need to be recompiled for each platform. However, compilers are platform-specific and require the program to be recompiled for each platform.
5. Security
- Compiled programs are more secure as the source code is not readily available in the executable. However, because the code is machine code, decompilation is possible using specialized programs. Interpreted programs are less secure as the source code is readily available and can be easily manipulated.
6. Error detection
- Interpreted programs detect errors more efficiently as compilation is not required. Therefore, the detection of syntax and semantic errors occurs during the runtime of the program. Compiled programs detect errors during compilation but do not include general error messages as interpreted programs do.
7. Resource Usage
- Compiled programs consume fewer resources (memory and CPU time) since they are pre-compiled. Interpreted programs consume more time and resources since they need to process the code.
In Conclusion
In summary, compilers and interpreters are software tools essential for translating high-level languages into machine code. While both tools achieve the same goal, they have significant differences in their usage, execution time, memory usage, debugging, portability, security, error detection, and resource usage.
While compiled programs are faster and more secure, they require more memory and are platform-specific. On the other hand, interpreters are more portable and more accessible to debug. It is up to the developer to choose the tool that best suits their needs based on the specified requirements of the project.