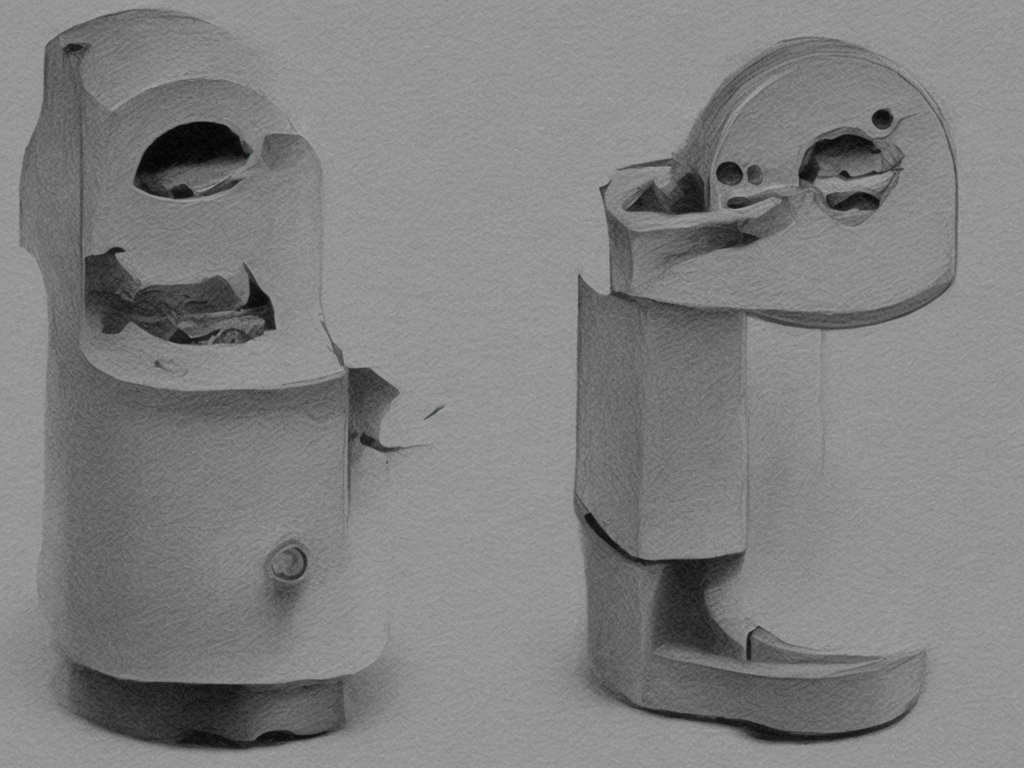
Constructors and destructors are two essential functions in object-oriented programming languages like C++, Java, and Python. Both these functions are used to create and destroy objects, respectively. However, many beginners in programming find it challenging to differentiate between constructors and destructors. In this article, we will discuss the difference between constructor and destructor, their working, and implementation.
Constructor:
A constructor is a special function that is automatically called when an object is created or instantiated. In other words, a constructor is a method that is used to initialize the object's data members or properties when it is created. The primary purpose of the constructor is to allocate the memory required for the object, set its initial state and values, and perform any additional tasks if necessary.
Constructors can be used to initialize the values of attributes, allocate resources, and perform any other initialization tasks. In C++, constructors have the same name as the class and do not have a return type. A constructor is executed only once when the object is created and not subsequently. In addition, there can be more than one constructor for a class, as long as they have different parameters.
Some of the key features of constructors are:
1. Name: Contrary to regular functions, constructors must have the same name as the class they belong to.
2. Return Type: Constructors do not have any return type, not even void.
3. Access Modifiers: Constructors can have access modifiers like public, private, or protected.
4. Parameters: Constructors can also receive parameters to initialize the attributes of the object.
5. Implicit: Constructors are called implicitly when an object of the class is created.
6. Single Execution: Constructors are executed only once during the lifetime of an object.
Destructors:
A destructor is a special function that gets called when the object is destroyed or deleted. The primary purpose of a destructor is to free up any resources that the object might have acquired during its lifetime, such as memory allocation. A destructor is the opposite of the constructor, as it gets called when the object is deleted or goes out of scope.
Like constructors, destructors are also named after the class, but with a tilde symbol (~) preceding the class name. Destructors also do not have any return type, not even void. Destructors are called automatically by the system, and there can be only one destructor for a class.
Some of the key features of destructors are:
1. Name: Destructors must have the same name as the class, with a tilde symbol (~) preceding it.
2. Return Type: Destructors do not have any return type, not even void.
3. No Parameters: Unlike constructors, destructors do not receive any parameters.
4. Single Execution: Destructors are also executed only once during the lifetime of an object.
5. Automatic: Destructors are called automatically by the system when an object is destroyed.
6. Maintain Object State: Destructors maintain the object's state before being destroyed.
Difference Between Constructor and Destructor:
1. Purpose: The primary purpose of a constructor is to initialize the object and allocate memory. In contrast, the primary purpose of a destructor is to release the memory and clean up any resources held by the object.
2. Name: Constructors have the same name as the class, while destructors have the same name but with a tilde symbol (~) preceding it.
3. Order of Execution: Constructors are executed when an object is created, while destructors are executed when an object is destroyed.
4. Return Type: Constructors do not have any return type, while destructors also do not have any return type, not even void.
5. Parameters: Constructors can have parameters while destructors cannot.
6. Object State: Constructors are used to initialize the object's state, while destructors are used to maintain the object's state before it is destroyed.
7. Multiple Definitions: A class can have more than one constructor with different parameters, but only one destructor.
Conclusion:
In summary, both constructors and destructors are essential functions in object-oriented programming languages. Constructors are used to create and initialize objects, while destructors are used to clean up and release resources held by an object. The main difference between constructors and destructors is their purpose and the order of execution. Constructors are executed when an object is created, while destructors are executed when an object is destroyed. A class can have multiple constructors, but only one destructor. It is crucial to understand the difference between constructors and destructors to write efficient and error-free code.