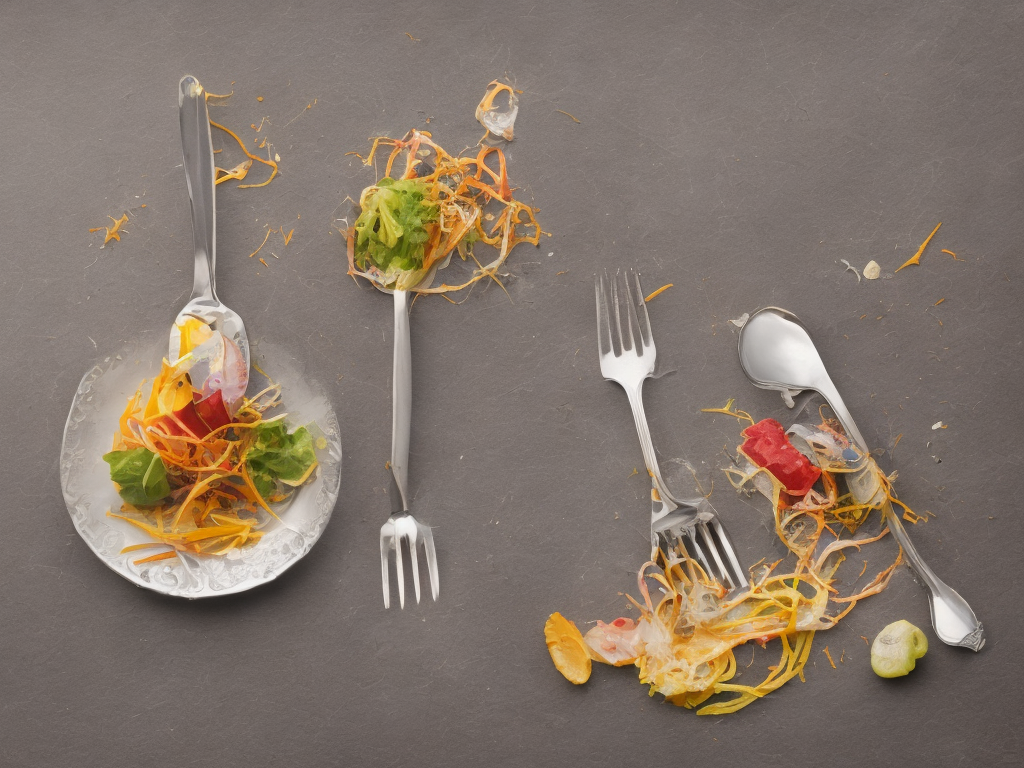
Introduction
Fork and vfork are two common concepts in Unix-based systems. They are both system calls that create new processes, but they differ in how they do it. Fork and vfork have distinct features which makes each one suitable for different use cases. Understanding the difference between fork and vfork is important for developers who work with Unix-based systems.
Fork
The fork system call creates a new process by duplicating the calling process. The new process, called the child process, is a copy of the parent process. The child process has its own memory space, file descriptors, and process ID. The child process executes the same code as the parent process from the point where the fork system call was made. The parent process continues executing from the same point after the fork system call returns. The child process is created with a copy of the parent process’s memory space. Any changes made to the memory space by the child process do not affect the parent process.
Fork is often used in concurrent programming, where multiple threads or processes work together to accomplish a task. Fork can be used to create multiple processes that share resources such as variables or file descriptors. Each process can execute a different portion of the code and communicate with each other. The fork system call is commonly used in Unix-based operating systems like Linux to create new processes.
Example of Fork System Call
The following code demonstrates how fork is implemented in C:
```
#include
#include
#include
int main() {
int pid = fork();
if (pid == -1) {
// Error handling
fprintf(stderr, "Failed to fork\n");
exit(EXIT_FAILURE);
} else if (pid == 0) {
// Child process
printf("I am the child process, my process ID is %d\n", getpid());
exit(EXIT_SUCCESS);
} else {
// Parent process
printf("I am the parent process, my child's process ID is %d\n", pid);
exit(EXIT_SUCCESS);
}
}
```
The code above creates a new process using fork. This process prints out its process ID and exits. If the child process is created successfully, its process ID is printed by the child process, and the parent process prints out the child's process ID. If an error occurs during the fork call, an error message is printed to stderr.
vfork
vfork is another system call used to create a new process. Unlike fork, vfork does not create a copy of the parent process. Instead, vfork creates a new process that shares the parent process’s memory space. The new process is also created in a suspended state, meaning it cannot run until it returns from the function that called vfork or calls execve.
vfork is used when a new process needs to be created with minimal overhead. Because vfork shares the parent process’s memory space, any changes made by the child process are visible to the parent process. This makes vfork suitable for cases where the new process needs to modify or read a large amount of data without duplicating it.
Example of vfork System Call
The following code demonstrates how vfork is implemented in C:
```
#include
#include
#include
int main() {
int pid = vfork();
if (pid == -1) {
// Error handling
fprintf(stderr, "Failed to vfork\n");
exit(EXIT_FAILURE);
} else if (pid == 0) {
// Child process
printf("I am the child process, my process ID is %d\n", getpid());
exit(EXIT_SUCCESS);
} else {
// Parent process
printf("I am the parent process, my child's process ID is %d\n", pid);
exit(EXIT_SUCCESS);
}
}
```
The code above creates a new process using vfork. This process prints out its process ID and exits. If the child process is created successfully, its process ID is printed by the child process, and the parent process prints out the child's process ID. If an error occurs during the vfork call, an error message is printed to stderr.
Differences between Fork and vfork
The following are the key differences between fork and vfork:
1. Memory sharing: Fork creates a copy of the parent process’s memory space for the child process, while vfork shares the parent process’s memory space with the child process.
2. Performance: Because fork creates a copy of the parent process’s memory space, it can be slower than vfork. Vfork creates a new process with minimal overhead since it does not copy the memory space.
3. Parent process behavior: In fork, the parent process continues to execute from the point where the fork system call was made. In vfork, the parent process is suspended until the child process either returns from the function that called vfork or calls execve.
4. Child process behavior: In fork, the child process is a copy of the parent process and has its own process ID. In vfork, the child process shares the parent process’s memory space and has the same process ID as the parent process.
Use Cases
The following are some typical use cases for fork and vfork:
1. Fork: When you need to create a new process that is a copy of an existing process and you want the two processes to be independent of each other, you should use fork. For example, you can use fork to create a new process to handle incoming network requests while the original process continues to listen for incoming requests.
2. Vfork: When you need to create a new process that needs to access the same memory space as the parent process and you want to avoid the overhead of copying the parent process’s memory space, you should use vfork. For example, you can use vfork to create a new process to perform a computationally intensive operation on a shared data structure.
Conclusion
Fork and vfork are two system calls used to create new processes in Unix-based systems. Fork creates a copy of the parent process’s memory space for the child process, while vfork shares the parent process’s memory space with the child process. Fork is typically used when you need to create a new process that is a copy of an existing process, while vfork is used when you need to create a new process that needs to access the same memory space as the parent process. Understanding the differences between the two system calls is important for developers who work with Unix-based systems.