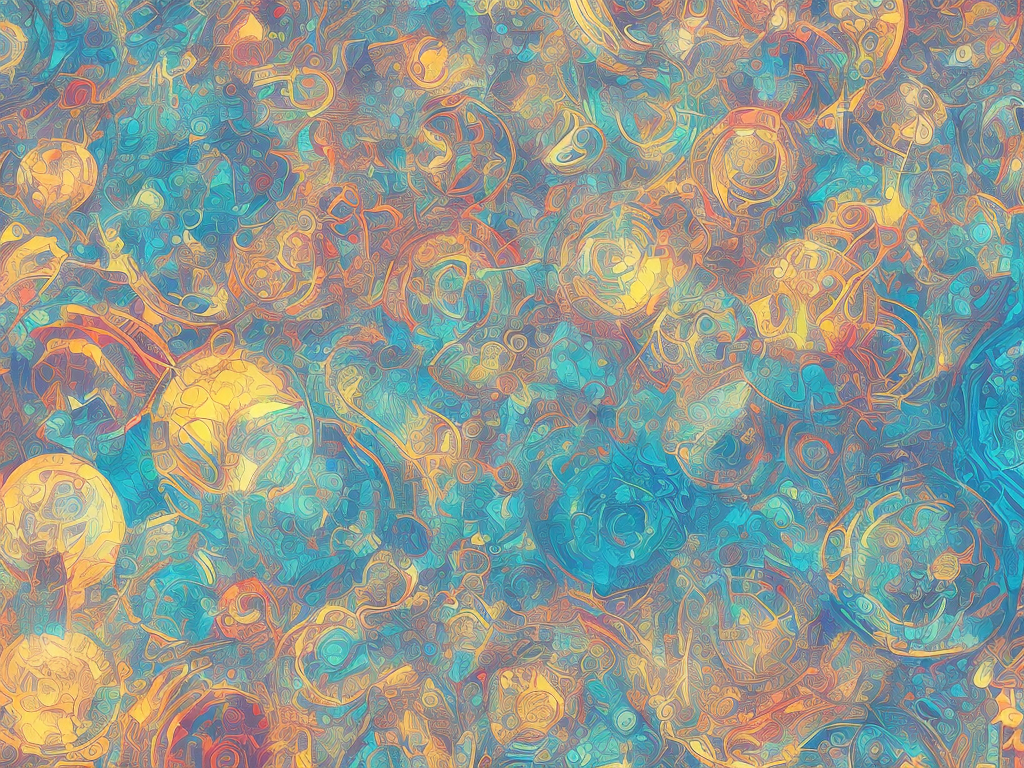
When it comes to object-oriented programming (OOP), there are two important concepts that are often used to define the structure and behavior of classes: interfaces and abstract classes. While they may seem similar, there are some key differences between the two that programmers need to understand in order to make the best use of them in their projects.
To begin with, an interface is a collection of abstract methods that define a contract for implementing classes. It provides a set of method signatures without any implementation details. In other words, an interface specifies what methods a class should have, but it doesn't provide any implementation for those methods.
On the other hand, an abstract class is a class that cannot be instantiated and is primarily used as a base class for deriving other classes. It can have both abstract and non-abstract methods. An abstract method is a method without any implementation, similar to the methods in an interface. However, an abstract class can also have implemented methods, which means it can provide a default behavior for certain methods.
One key difference between interfaces and abstract classes is that a class can implement multiple interfaces, but it can only inherit from one abstract class. This is known as multiple inheritance through interfaces, and it allows a class to define its behavior based on multiple contracts specified by different interfaces. For example, a class can implement interfaces such as "Drawable" and "Movable" to indicate that it can be drawn on a screen and can be moved around.
On the other hand, the restriction of single inheritance with abstract classes means that a class can only inherit behaviors and structures from its parent abstract class. This can be seen as a limitation when compared to interfaces, but it also allows for a more structured and hierarchical class design.
Another difference is that an abstract class can have fields (member variables) and properties, while an interface can only have constants. A field in an abstract class can hold data that is common to all derived classes, whereas an interface cannot define any state.
Additionally, abstract classes can provide a default implementation for methods, making them optional to implement in the derived classes. This allows for a common implementation to be shared among multiple derived classes, while still providing the flexibility to override the default behavior when needed. Interfaces, on the other hand, do not allow for default implementations. All methods defined in an interface must be implemented by the class that implements it.
In terms of usage, interfaces are often used to define behavior contracts that allow different classes to work together in a unified way. They provide a level of abstraction that allows for loose coupling and flexibility in selecting which specific classes to use in a given situation. For example, an interface called "Sortable" can be implemented by different classes to indicate that they can be sorted using a specific sorting algorithm.
On the other hand, abstract classes are used when there is a need for a common base class that provides some default behavior and state. They are often used to define a common interface and partial implementation for a set of related classes. For example, an abstract class called "Animal" can define common methods such as "eat" and "sleep", which can be inherited and overridden by its derived classes like "Dog" and "Cat".
In terms of extensibility, interfaces provide a more flexible approach. Since a class can implement multiple interfaces, it can be easily extended to support new behaviors by implementing additional interfaces. On the other hand, extending an abstract class requires creating a new derived class, which can be more rigid and less flexible.
Another important difference is that interfaces are used for achieving polymorphism, which is the ability to treat objects of different classes in a uniform way. By programming to interfaces, you can write code that is more generic and reusable, as it can work with any object that implements a specific interface. This is particularly useful when creating libraries or frameworks that need to interact with various classes.
Abstract classes, on the other hand, are more suitable for creating a hierarchy of related classes where certain behaviors and structures are shared among them. They provide a way to define a common base class that can be specialized by derived classes.
In summary, the main differences between interfaces and abstract classes lie in their purpose, usage, and flexibility. Interfaces are used to define behavior contracts and achieve polymorphism, while abstract classes are used as base classes and provide a default implementation. Interfaces allow multiple inheritance and are more extensible, while abstract classes provide a structured class hierarchy and allow for sharing of common behavior and state. Both interfaces and abstract classes have their own advantages and should be chosen based on the specific requirements and design considerations of the project at hand.