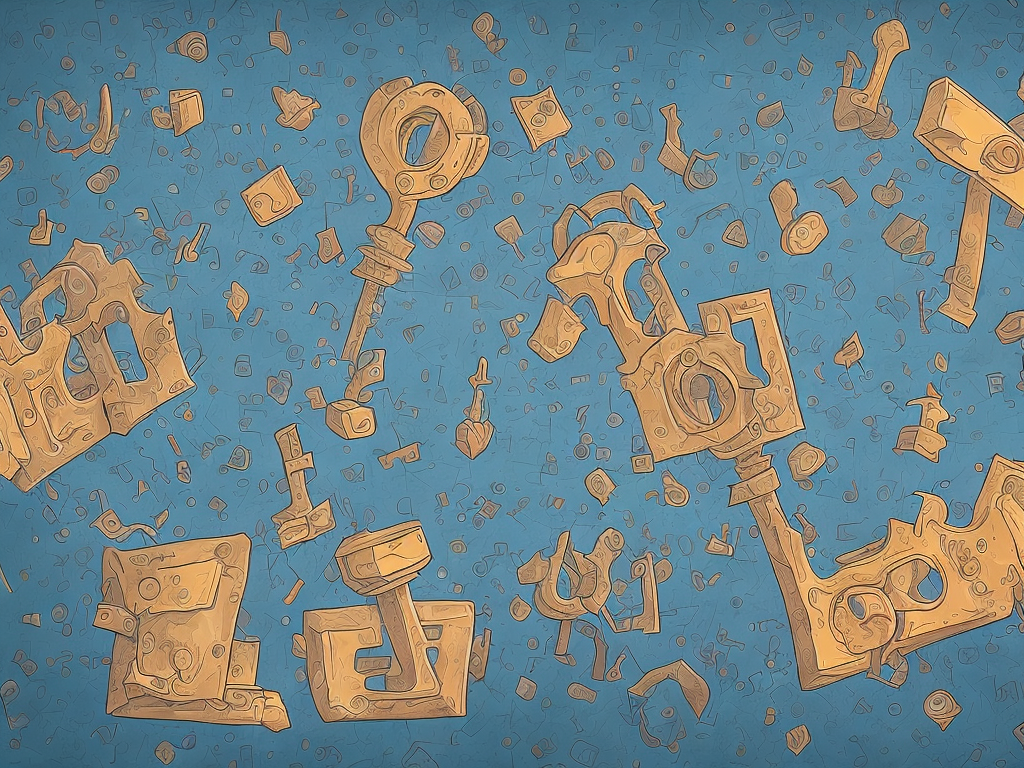
Lists and tuples are two of the most commonly used data structures in Python and other programming languages. While they may seem similar at first glance, there are some key differences between the two. In this article, we will explore the main differences between lists and tuples in Python.
Definition
A list is a collection of elements that is ordered and changeable. Lists are represented by square brackets [] and elements are separated by commas. Lists are mutable, which means they can be changed after creation.
A tuple is also a collection of elements that is ordered, but unlike lists, tuples are immutable. Tuples are represented by parentheses () and elements are similarly separated by commas.
Mutability
One of the most significant differences between lists and tuples is their mutability. As mentioned, lists are mutable, which means that they can be changed, modified, or extended after they are created. For example, we can add new elements to a list, delete existing elements, or change the value of an element.
On the other hand, tuples are immutable, which means that they cannot be modified once they are created. Once a tuple is created, you cannot add, delete or change any element in it. If we try to change the value of an element in a tuple, we get a TypeError.
For example:
```python
# list example
>>> my_list = [1, 2, 3, 4, 5]
>>> my_list[2] = 6
>>> print(my_list)
[1, 2, 6, 4, 5]
# tuple example
>>> my_tuple = (1, 2, 3, 4, 5)
>>> my_tuple[2] = 6
TypeError: 'tuple' object does not support item assignment
```
Length
Another difference between lists and tuples is their length. Lists can be of any length, and their size can change dynamically as elements are added or removed from the list. On the other hand, tuples are fixed in size, meaning that they have a fixed number of elements once they are created.
For example:
```python
>>> my_list = [1, 2, 3, 4, 5]
>>> print(len(my_list))
5
>>> my_tuple = (1, 2, 3, 4, 5)
>>> print(len(my_tuple))
5
```
Uses
Lists and tuples have different use-cases. Lists are best suited for situations where we need to store a collection of items that may change over time. With lists, we can add, remove or modify any element in the list at any time. Lists are used for holding large collections of data such as user data, product data, and many others.
Tuples, on the other hand, are used in cases where we need to store a collection of items that will not change. Tuples can be used to assign multiple values to different variables in one line of code. They are also used to return multiple values from a function. When we have functions that return multiple values, tuples come in handy since we can pack all the values into a tuple and return them as one object.
For example:
```python
def get_name_and_age():
name = "Alice"
age = 25
return name, age
# use a tuple to store the returned values
name_age_tuple = get_name_and_age()
print(name_age_tuple[0]) # Alice
print(name_age_tuple[1]) # 25
```
Performance
Performance-wise, tuples are faster than lists. Since tuples are immutable, they require less memory and are faster to access than lists. In contrast, lists are slower than tuples because they require more memory and time to access their elements.
For example, let's compare the time it takes to access the second element in a list and a tuple with 1 million items each:
```python
import time
my_list = list(range(1000000))
my_tuple = tuple(range(1000000))
start_list = time.time()
elem_list = my_list[1]
end_list = time.time()
start_tuple = time.time()
elem_tuple = my_tuple[1]
end_tuple = time.time()
print("Time to access second element in list: ", end_list - start_list)
# Time to access second element in list: 5.0067901611328125e-06
print("Time to access second element in tuple: ", end_tuple - start_tuple)
# Time to access second element in tuple: 4.76837158203125e-07
```
As we can see from this example, accessing an element in a tuple is faster than accessing an element in a list.
Conclusion
In conclusion, the main difference between lists and tuples is their mutability. Lists are mutable, while tuples are immutable. Lists can be of any length and their size can change at any time, whereas tuples have a fixed size and cannot be modified after creation. Lists are used for situations where we need to store a collection of items that may change over time, while tuples are used in cases where we need to store a collection of items that will not change. Tuples are faster than lists since they require less memory and are faster to access.