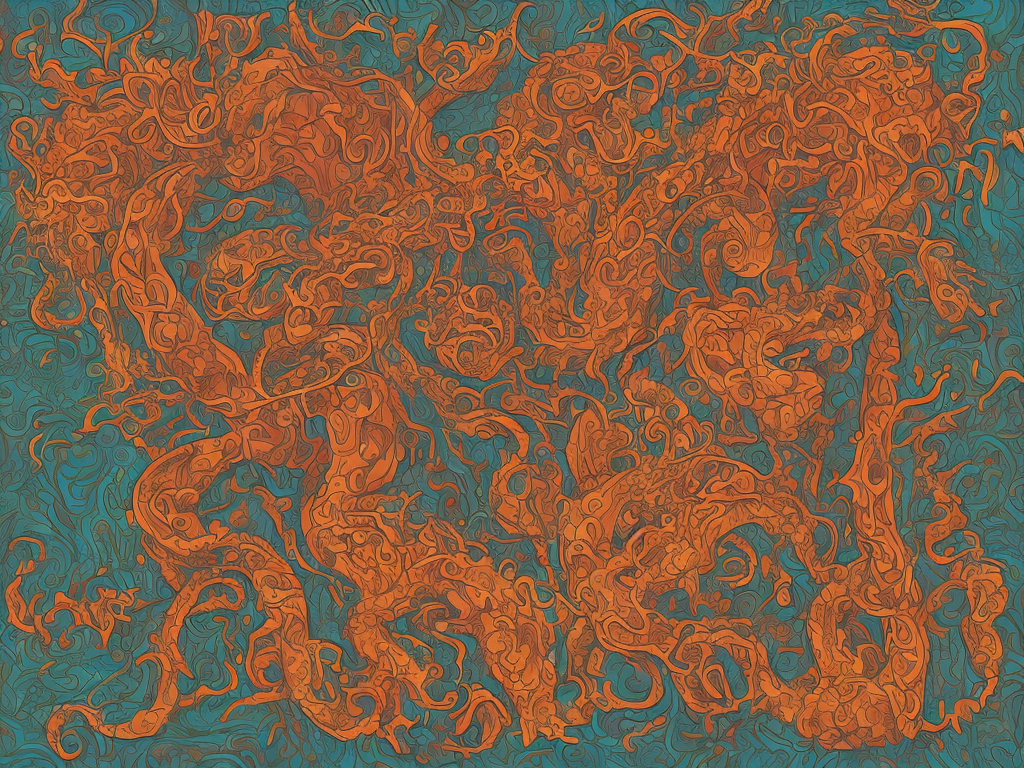
As a developer or data analyst, you may find yourself manipulating data frames in Pandas. Two of the most frequently used methods for accessing elements within a Pandas data frame are .loc and .iloc. In order to fully utilize Pandas data frames, it is important to understand the differences between these two methods.
Let’s start with a brief overview of what Pandas data frames are. Data frames in Pandas are two-dimensional, size-mutable, and tabular data structures that are commonly used in data processing tasks. They can be thought of as tables that have rows and columns, with each column containing values of a specific data type.
.loc stands for label-location, and it is used to access data in a data frame by label. Essentially, .loc is used to access data using the row and column labels. In order to use .loc, you must have the specific row and column labels.
On the other hand, .iloc stands for integer-location, and it is used to access data by position or index rather than by label. .iloc is used to access data using the row and column index positions.
One of the major differences between .loc and .iloc is the way they handle slicing. When using .loc, both the start and end indices are included in the slice. For example, if you had a data frame with labels A through E, and you wanted to select all rows from A to C, you would use the following syntax:
```python
df.loc['A':'C']
```
On the other hand, .iloc behaves differently when using slicing. When using .iloc, the start index is included, but the end index is excluded. For example, if you had a data frame with four rows, and you wanted to select the first three rows, you would use the following syntax:
```python
df.iloc[0:3]
```
Another key difference between .loc and .iloc is the type of input they accept. .loc accepts data frame labels, and works with both rows and columns. This allows you to select specific rows and specific columns at the same time. For example, if you had a data frame with columns ‘Name’, ‘Age’, and ‘Gender’, and you wanted to select the rows where the age was greater than 30 and only the ‘Name’ and ‘Age’ columns, you would use the following syntax:
```python
df.loc[df['Age'] > 30, ['Name', 'Age']]
```
On the other hand, .iloc only accepts integer inputs. This means that you cannot use column labels when selecting columns. If you wanted to select specific columns using .iloc, you would need to use integer positions instead. For example, if you had a data frame with the columns ‘Name’, ‘Age’, and ‘Gender’, and you wanted to select only the first two columns, you would use the following syntax:
```python
df.iloc[:, 0:2]
```
As previously mentioned, .loc is used to access data using the row and column labels. This means that .loc is typically used when working with data frames that have explicitly labeled rows and columns. This can be useful when working with datasets that have unique identifiers for rows, and column names that accurately describe the data contained within the column.
On the other hand, .iloc is typically used when working with data frames where the row and column labels may not have any meaning or significance. This can be useful when working with data frames that do not have explicit row or column labels, and where their order may be more important than their label or identifier.
One other important difference between .loc and .iloc is how they handle changes made to the data frame. When data is filtered or otherwise manipulated using .loc, any changes made to the resulting data frame will be reflected in the original data frame. This is because changes made to the filtered data frame are actually changing the underlying data in the original data frame.
On the other hand, when data is filtered or otherwise manipulated using .iloc, any changes made to the resulting data frame will not be reflected in the original data frame. This is because changes made to the filtered data frame are actually creating a new data frame, rather than changing the underlying data in the original data frame.
In conclusion, .loc and .iloc are both important methods for accessing and manipulating data within Pandas data frames. The major differences between the two methods revolve around how they handle slicing, the type of input they accept, and how they handle changes made to the data frame. Understanding these differences can help you to better utilize Pandas data frames in your data processing tasks.