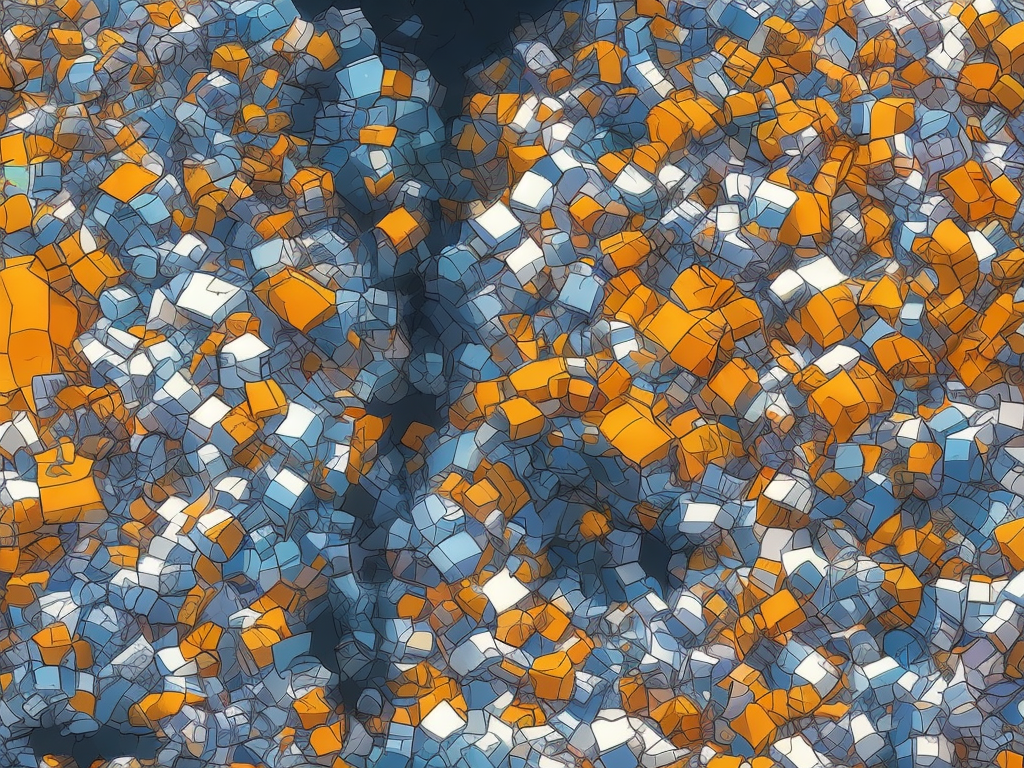
Overloading and overriding are two important concepts in object-oriented programming that allow developers to create more flexible and efficient code by reusing existing methods. Both techniques involve methods in classes, but there are some crucial differences between overloading and overriding that developers need to understand in order to use them effectively.
Overloading:
Overloading is a technique in which a class has multiple methods with the same name but different parameters. This allows developers to provide alternative ways of calling a method based on different data types or numbers of arguments. When a method is overloaded, the compiler determines which version of the method to invoke based on the type and number of arguments passed to it.
Let's take an example to better understand overloading. Consider a class called Calculator with two methods: add and addMany. The add method takes two integers as arguments and returns their sum, while the addMany method takes a variable number of arguments and returns the sum of all the numbers passed in.
```java
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public int addMany(int... numbers) {
int sum = 0;
for (int num : numbers) {
sum += num;
}
return sum;
}
}
```
In this example, we have overloaded the add method. If we call the add method with two integer arguments, the compiler will invoke the add method that accepts two integers and returns their sum. On the other hand, if we call the add method with more than two integer arguments, the compiler will invoke the addMany method and return the sum of all the numbers.
Overriding:
Overriding, on the other hand, is a concept that allows a subclass to provide its own implementation of a method that is already defined in its superclass. This feature is essential for achieving polymorphism, where multiple objects of different classes can be treated as objects of a common superclass.
To override a method, the subclass must have the same method signature (same name, same return type, and same parameters) as the superclass method. By doing so, the subclass can replace the behavior of the superclass method without modifying the existing code.
Let's consider an example to demonstrate overriding. Suppose we have a class called Animal with a method called makeSound:
```java
public class Animal {
public void makeSound() {
System.out.println("The animal makes a sound");
}
}
```
Now, let's create a subclass called Dog that extends the Animal class and overrides the makeSound method:
```java
public class Dog extends Animal {
@Override
public void makeSound() {
System.out.println("The dog barks");
}
}
```
In this example, the Dog class overrides the makeSound method from the Animal class and changes the behavior of the method. When we create an instance of the Dog class and call the makeSound method, it will print "The dog barks" instead of "The animal makes a sound," which is the behavior defined in the Animal class.
Key Differences between Overloading and Overriding:
1. Definition:
Overloading is a technique where a class has multiple methods with the same name but different parameters. Overriding, on the other hand, is a concept that allows a subclass to provide its own implementation of a method that is already defined in its superclass.
2. Inheritance:
Overloading is resolved during compile-time and does not involve inheritance. Overriding, on the other hand, is resolved during runtime and requires inheritance.
3. Method Signature:
In overloading, the methods must have the same name but different parameters, while in overriding, the methods must have the same signature (same name, same return type, and same parameters).
4. Invocation:
Overloaded methods are invoked based on the type and number of arguments passed to them at compile-time. Overridden methods, on the other hand, are invoked based on the type of the object at runtime.
5. Return Types:
Overloaded methods can have different return types as long as the parameters differ. In overriding, the return type must be the same or a subtype of the return type of the overridden method.
6. Scope:
Overloaded methods can be in the same class or different classes within the same package. Overridden methods, however, must be in a subclass and have the same visibility or a wider visibility than the overridden method.
In conclusion, overloading and overriding are two fundamental concepts in object-oriented programming that allow developers to create more flexible and efficient code. Overloading provides multiple ways to call a method based on different parameters, while overriding allows a subclass to provide its own implementation of a method defined in its superclass. Understanding the differences between overloading and overriding is crucial for writing concise and maintainable code in object-oriented programming languages.