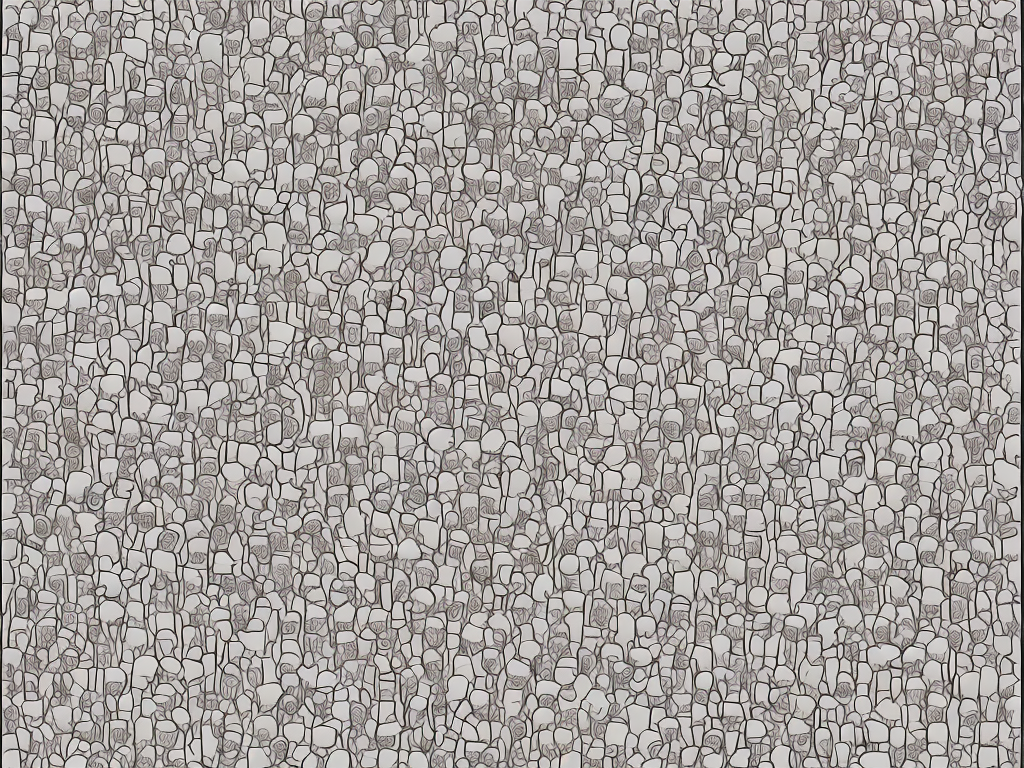
Stacks and queues are data structures that are used to organize and manipulate data in computer programs. In some cases, they can be used interchangeably, but in other cases, the choice of a suitable data structure can have a significant impact on the performance and the correctness of the code. In this article, we will explain the difference between stacks and queues, their properties, and their applications.
Stacks
A stack is a collection of elements that supports two primary operations: adding an element to the top, and removing an element from the top. The order of insertion and removal of elements follows the principle of Last-In-First-Out (LIFO), which means that the last element added to the stack is the first one that is removed. Stacks can be implemented using arrays or linked lists.
The operation to add an element to the top of a stack is called push, while the operation to remove an element from the top is called pop. The push operation adds an element to the top of the stack, while the pop operation removes the top element from the stack and returns its value. If the stack is empty and the pop operation is called, a stack underflow error occurs. Similarly, if the stack is full and the push operation is called, a stack overflow error occurs.
Stacks are mostly used in situations where the order of operations is important, and the most recent operation needs to be processed first. For example, when parsing mathematical expressions, a stack can be used to store the operators and operands in the order in which they appear in the string. When an operator is encountered, it can be popped from the stack, and the operands can be evaluated. Another example is in the implementation of recursive algorithms, which require a stack to keep track of the function calls and their corresponding variables.
Queues
A queue is a collection of elements that supports two primary operations: adding an element to the rear, and removing an element from the front. The order of insertion and removal of elements follows the principle of First-In-First-Out (FIFO), which means that the first element added to the queue is the first one that is removed. Queues can be implemented using arrays or linked lists.
The operation to add an element to the rear of a queue is called enqueue, while the operation to remove an element from the front is called dequeue. The enqueue operation adds an element to the rear of the queue, while the dequeue operation removes the front element from the queue and returns its value. If the queue is empty and the dequeue operation is called, a queue underflow error occurs. Similarly, if the queue is full and the enqueue operation is called, a queue overflow error occurs.
Queues are mostly used in situations where the order of arrival of elements is important, and the oldest element needs to be processed first. For example, when simulating a network of interconnected computers, a queue can be used to store the packets of data that need to be transmitted. The packets are added to the rear of the queue as they arrive and are removed from the front of the queue when they are transmitted. Another example is in the implementation of BFS (Breadth-First Search) algorithms, which require a queue to store the nodes that need to be visited in the order of their distance from the starting node.
Difference between Stacks and Queues
The primary difference between stacks and queues is the order in which the elements are inserted and removed. Stacks follow the LIFO (Last-In-First-Out) principle, while queues follow the FIFO (First-In-First-Out) principle. This fundamental difference affects the choice of the data structure based on the requirements of the problem.
Another difference is the set of operations that each data structure supports. Stacks support push and pop operations, while queues support enqueue and dequeue operations. Both data structures also support a few additional operations, such as peek (to retrieve the top or front element without removing it), size (to obtain the number of elements in the data structure), and isEmpty (to check if the data structure is empty).
Stacks are more suitable for situations where the ordering of elements is important and where the recent elements need to be processed first. Examples include parsing mathematical expressions, implementing recursive algorithms, and simulating call stacks in programs. Queues are more suitable for situations where the ordering of elements is important, and where the oldest elements need to be processed first. Examples include simulating networks, implementing BFS algorithms, and processing requests in a web server.
Conclusion
Stacks and queues are two fundamental data structures that have different properties and applications. Stacks follow the LIFO (Last-In-First-Out) principle, while queues follow the FIFO (First-In-First-Out) principle. Both data structures support push and pop (or enqueue and dequeue) operations, as well as peek, size, and isEmpty operations. The choice of a suitable data structure depends on the requirements of the problem and the ordering of the elements. By understanding the difference between stacks and queues, programmers can write more efficient and correct code for a wide range of applications.