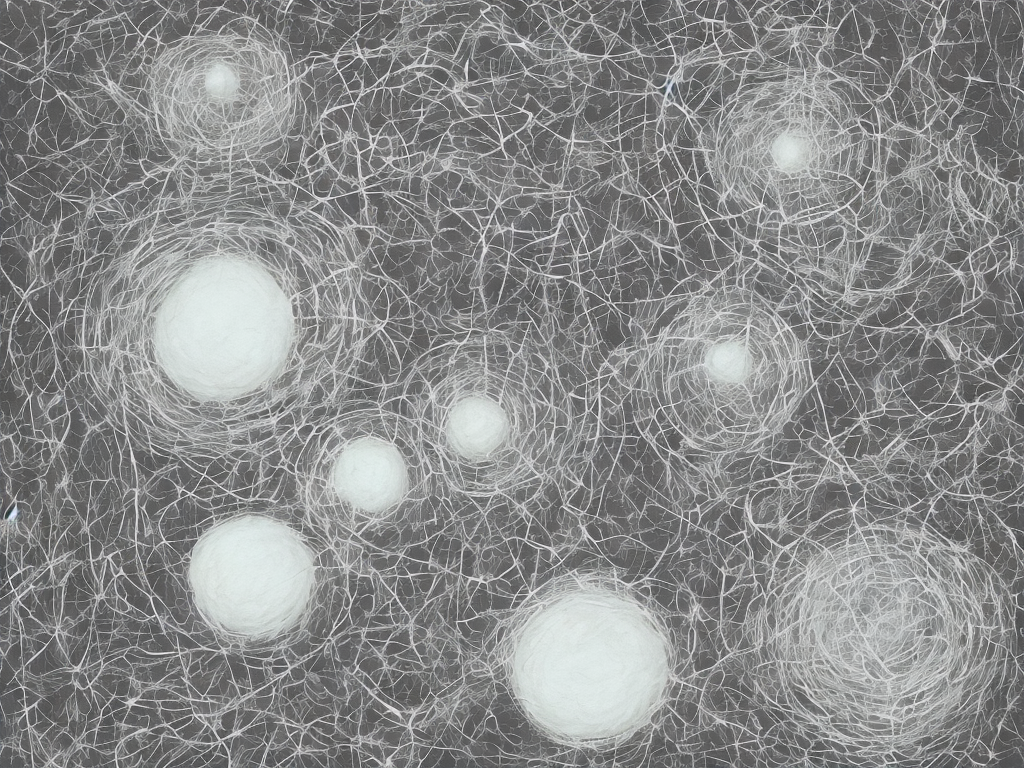
Hashing is an important concept in computer science, particularly in the field of databases. It involves mapping data elements to memory locations through the use of a hash function. There are two main types of hashing, static and dynamic. This article will explain the difference between the two.
Static Hashing
Static hashing, also known as fixed hashing, involves a fixed number of hash buckets that are used to store elements. Each hashmap has a fixed size and cannot be resized during runtime. In this type of hashing, the number of buckets remains constant, even if the number of elements changes.
Static hashing has several advantages compared to dynamic hashing. Firstly, it is usually faster because the hash functions are simpler and fixed, and there is no need to resize or reorganize the hashmap during runtime. Secondly, it is easier to implement because the fixed size of the hashmap means that there are no complex dynamic data structures required. Finally, the use of a static hashmap is more predictable, as the number of elements and buckets is fixed, meaning it is easier to estimate the amount of memory required.
However, there are also several disadvantages to static hashing. For example, if the number of elements is much smaller than the number of hash buckets, then there will be a lot of empty buckets in the hashmap, which wastes memory. On the other hand, if there are more elements than there are hash buckets, collisions are likely to occur, causing an increase in search time. Therefore, it is important to optimize the hash function so that the number of collisions is minimized.
Dynamic Hashing
Dynamic hashing is a more flexible method of hashing that can adjust the size of the hashmap based on the number of elements. This means that the number of buckets can be increased or decreased during runtime, allowing for better memory management. In dynamic hashing, if the number of elements increases beyond the capacity of the hashmap, new buckets are added in order to accommodate the new elements. Conversely, if the number of elements decreases significantly, the number of buckets can be reduced in order to save memory.
The process of increasing or decreasing the size of the hashmap is called rehashing. Rehashing can be computationally expensive, especially if a lot of elements are involved. Therefore, it is important to optimize the rehashing process so that it occurs as infrequently as possible.
Dynamic hashing has several advantages over static hashing. Firstly, it is more memory-efficient because it can adjust the number of buckets based on the number of elements, reducing the number of empty buckets. Secondly, it can handle a variable number of elements, making it more versatile. Thirdly, dynamic hashing reduces the likelihood of collisions, leading to faster search times.
However, dynamic hashing also has several disadvantages. Firstly, it is more complex to implement, requiring more complex data structures and algorithms. Secondly, it has a higher computational cost due to the need for rehashing. Finally, it can be difficult to predict the amount of memory required by a dynamic hashmap, as it can change during runtime.
Conclusion
In conclusion, both static and dynamic hashing have their advantages and disadvantages. Static hashing is faster, easier to implement, and more predictable, but can waste memory and suffer from collisions if the number of elements is not correctly estimated. Dynamic hashing is more versatile, memory-efficient, and has a reduced likelihood of collisions, but is more complex to implement, computationally expensive, and difficult to predict the amount of memory that may be required. It is up to individual programmers and computer scientists to determine which type of hashing best suits their needs.