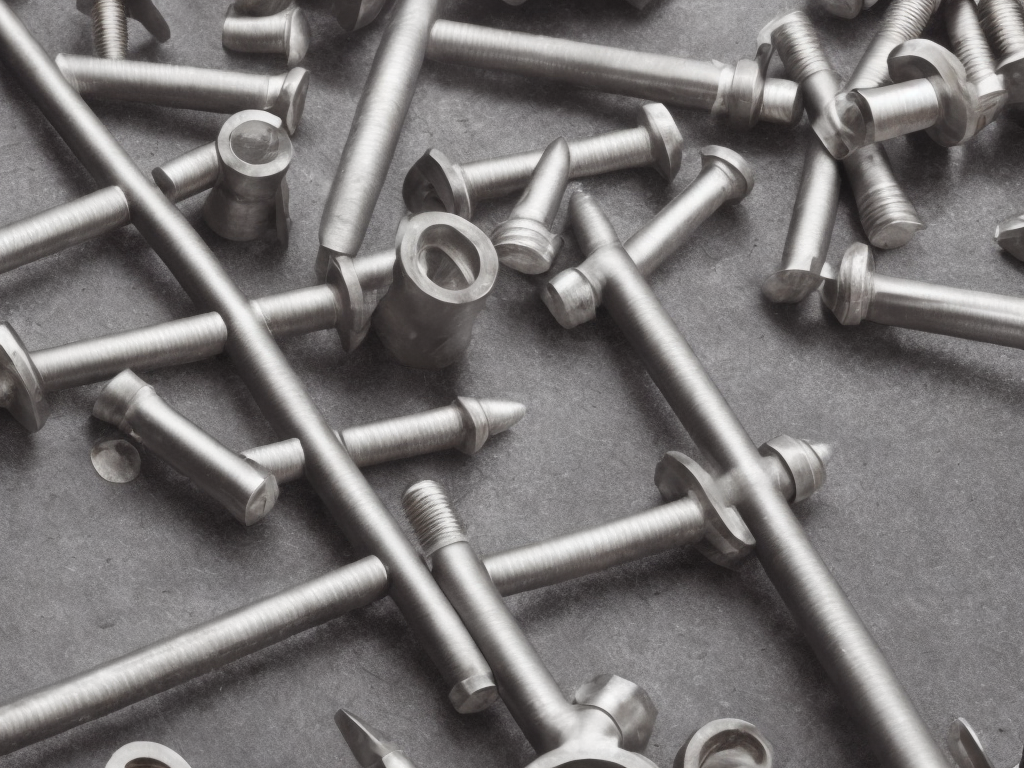
In the C programming language, two essential data types are available that help developers manipulate data structures. These two data types are known as Union and Structure. Both Union and Structure have different functionalities and are used in different scenarios.
Before diving in, it’s essential to understand data types. In computer science, a data type is an attribute of data which defines the range of values that can be stored by a variable. The fundamental data types used in C are integer, character, and float.
Now let's talk about the difference between these two data types.
Union:
A union is a user-defined data type that enables storage of different data types in a single memory location. In simple words, a union allows us to use the same memory space to store various data types, but we can access only one member at a time. It is similar to a structure, but the main difference is in the allocation of memory.
Memory is allocated enough to hold the member with the largest size, allowing different members inside a union to access the same memory space. One of the primary benefits of using a union is that you can conserve memory.
This image shows how the structure and the union variables allocate memory in the memory space:
The union variables are assigned a common memory address; thus, if we modify one union variable's value, the value of another variable gets changed as well.
Let's see the syntax and examples of the union:
Syntax:
uniondatatype
{
member1;
member2;
member3;
.
.
.
memberN;
};
Example:
union number
{
int num1;
float num2;
char num3;
};
int main()
{
union number n;
n.num1 = 10;
printf("num1: %d\n", n.num1);
n.num2 = 15.5;
printf("num2: %f\n", n.num2);
n.num3 = 'c';
printf("num3: %c\n", n.num3);
return 0;
}
Output:
num1: 10
num2: 15.500000
num3: c
The output shows that even after changing the value of a variable inside the union, it doesn't change the memory values of other variables; instead, it updates the same memory address for each variable.
Structure:
A structure is a user-defined data type that is a collection of related data members under a single name. It can store different data types in a single entity, which can be then accessed through various variables. The memory allocation for a structure is sequential, enabling each member to store its memory space independently.
The members in a structure can have different sizes and data types, unlike arrays, where all elements must have the same data type. Structures are useful in organizing multiple variables under one name that makes the code more readable.
Let's see the syntax and examples of the structure:
Syntax:
struct datatype
{
member1;
member2;
member3;
.
.
.
memberN;
};
Example:
struct book
{
char title[50];
char author[50];
int pages;
float price;
};
int main()
{
struct book b1 = {"C Programming", "Dennis Ritchie", 342, 320.05};
printf("Title: %s\n", b1.title);
printf("Author: %s\n", b1.author);
printf("Pages: %d\n", b1.pages);
printf("Price: %f\n", b1.price);
return 0;
}
Output:
Title: C programming
Author: Dennis Ritchie
Pages: 342
Price: 320.050000
This output displays values of structure members assigned to the structure variable.
So, what is the difference between Union and Structure?
1. Memory Allocation:
In structure, memory is allocated sequentially, and each member is assigned a separate memory location. On the other hand, in union, memory is allocated to accommodate the largest member size only.
2. Accessing the Members:
In structure, each member is accessed explicitly through its name or use the pointer to access the members, and all members store different values at different memory locations. But, in a Union, only one member is accessible at a given time, and the same memory location is referred to access different members at different times.
3. Size:
This is one of the most significant differences between a structure and a union because a structure allocates memory to each variable independently, whereas a union allocates memory to the variable based on the memory size of the most significant variable in the group. Therefore, the size of the Union ends up being smaller than the size of the structure.
4. Usage:
Structures are used when there is a need to store multiple elements under one name. Each member in the structure could hold a unique set of data, and each variable could use its memory space assigned independently. Unions, on the other hand, works when there is a need to store single data with varying data types.
Conclusion:
A structure and a union in C serve different purposes, but played a significant role in any program. Structures are preferred for storing multiple elements of different data types, while Unions are preferred when a single data element can be represented by multiple data types. Both structures and unions have disadvantages and advantages, so choosing the right data type for any given structure is a vital consideration. Understanding the difference between the union and structure is vital for C developers to apply them in their preferred programing scenario.