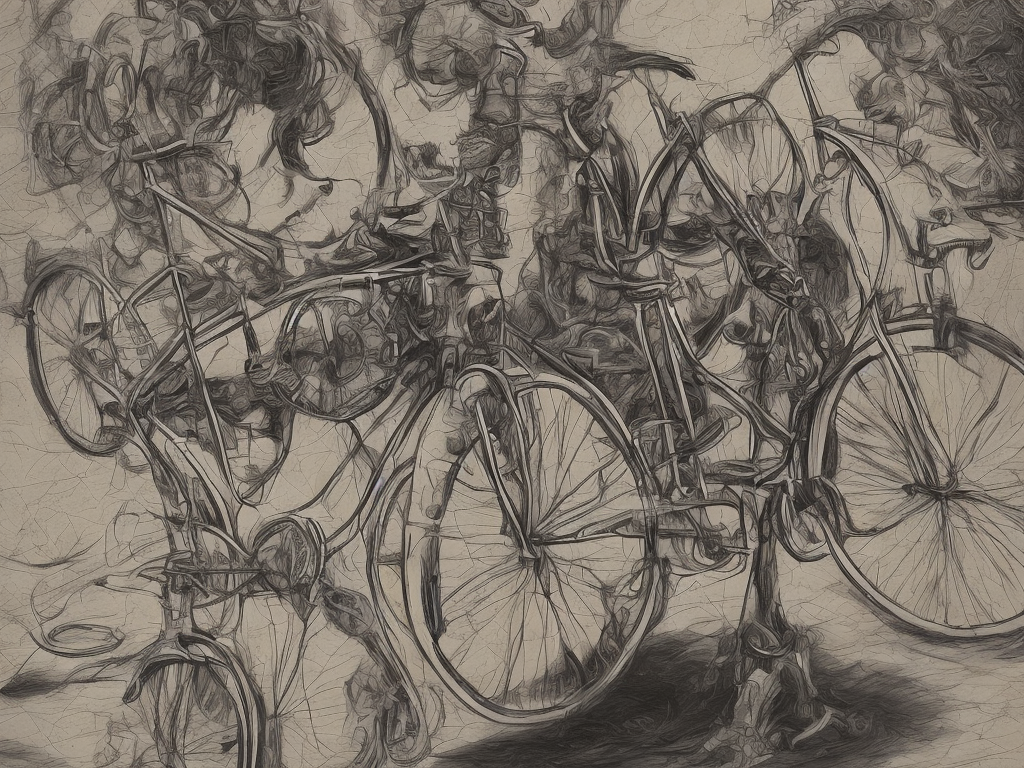
When it comes to programming, loops are a common feature of many languages. A loop allows a set of instructions to be executed repeatedly until a specific condition is met. Two types of loops commonly used in programming are the 'while' loop and the 'do-while' loop. Though both of these loops are used for the same purpose and have many similarities, there are some fundamental differences between them.
What is a while loop?
A while loop is a control flow statement that allows repeatedly executing a block of code as long as the condition is true. A while loop evaluates the condition before executing the block of code. If the condition is false, the loop will never be executed. While loops are commonly used when the number of iterations is unknown until runtime. The syntax for a while loop is as follows:
```
while (condition) {
// code to be executed
}
```
Here, the 'condition' is a logical expression that often makes use of relational or logical operators to compare values or conditions to determine whether the loop should continue or terminate.
What is a do-while loop?
A do-while loop is also a control flow statement that allows repeatedly executing a block of code until the condition is false. However, the primary difference between a while loop and a do-while loop is that a do-while loop executes the code block at least once, even if the condition is false. A do-while loop evaluates the condition after executing the code block. The syntax of a do-while loop is as follows:
```
do {
// code to be executed
} while (condition);
```
Here, the code block is executed first, and then the condition is checked to see if the loop needs to continue or terminate.
Let's understand the differences between a while loop and a do-while loop in detail.
1. The first difference between a while and do-while loop is their condition evaluation. In the while loop, the condition is checked first, and then the block of code is executed. However, in a do-while loop, the code block is executed first and then the condition is checked. In other words, the condition in a do-while loop is always evaluated at least once, even if the condition is false.
2. The second difference between the two is that a do-while loop executes the code block at least once in all situations. On the contrary, a while loop will not execute the block of code if the condition is initially false.
3. The third difference between the two is their syntax. In a while loop, the condition is placed in parentheses after the 'while' keyword. In contrast, the condition in a do-while loop is placed after the code block, following the 'while' keyword. This makes the do-while loop a little more readable.
4. The fourth difference is that a while loop is a pre-test loop, which checks for the condition before executing the code block. On the other hand, a do-while loop is a post-test loop that executes the code block once, and then checks for the condition.
5. The fifth difference is the scope of the variables in the loop. The scope of while loop variables is confined to the loop itself, whereas, the scope of variables used in a do-while loop extends beyond the loop. This is because, in the case of a do-while loop, the variables have already been declared before executing the code block.
6. The sixth difference is the total number of iterations. A while loop may or may not execute the code block at all if the condition is not met. On the other hand, a do-while loop will execute the code block at least once, irrespective of the condition.
When to use a while loop
While loops are ideal when the total number of repetitions is unknown, and the execution of the desired code depends on reaching a specific condition, such as user input. Additionally, if the code block is not required to be executed at all and can be skipped when the condition is initially false, a while loop is preferable.
When to use a do-while loop
Do-while loops are preferable when we require the code block to execute at least once, no matter what the condition may be. Additionally, if the code block requires user input and the loop terminates only when the condition is false, a do-while loop is a better choice.
Conclusion
While loops and do-while loops are both essential tools when it comes to writing efficient code. Both loops are used for the similar purpose to execute specific code multiple times until a specific condition is met. However, they have their fundamental differences. The while loop executes the code block only if the condition is true, whereas the do-while loop executes the block of code at least once, irrespective of the condition. It's essential to understand the differences between these two loop types so that programmers can choose the most appropriate loop based on their requirements.