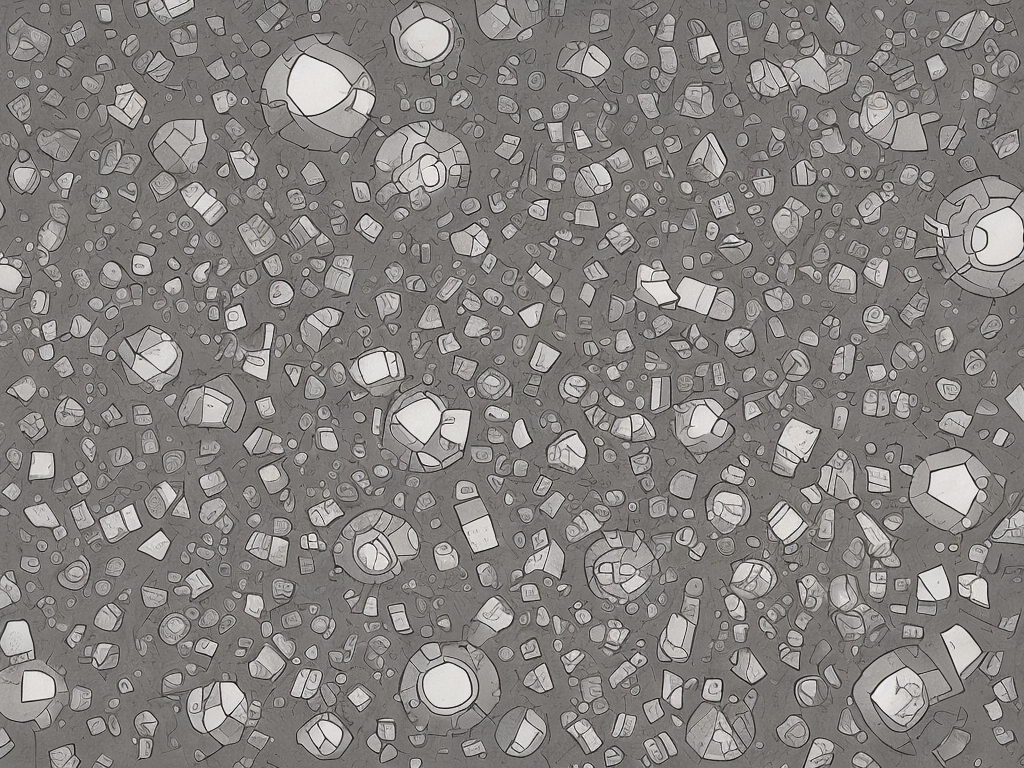
Find the Minimum Absolute Difference Between Two Elements in the Array That Are at least X Indices Apart
In computer science and data analysis, there are countless scenarios where finding the minimum absolute difference between elements in an array is necessary. However, in some cases, it becomes more complex when we have to consider a minimum distance or interval between the selected elements. In this article, we will explore an algorithm to solve the problem of finding the minimum absolute difference between two elements in an array that are at least X indices apart.
To truly understand the problem, let's first define the terms involved. An array, in the context of computer programming, is a data structure that holds a fixed number of elements of the same type. The absolute difference between two elements is the positive difference between them, regardless of their order. Lastly, the indices refer to the position of each element within the array, with the starting index typically being 0.
Let's consider an example to illustrate the problem. Suppose we have an array [5, 7, 10, 3, 12, 16, 11] and we want to find the minimum absolute difference between two elements that are at least 2 indices apart. In this case, the minimum absolute difference would be between 5 and 11, resulting in an absolute difference of 6.
To solve this problem efficiently, we can follow a simple algorithmic approach. First, we need to initialize a variable, let's call it minDiff, to store the minimum absolute difference. We can set its initial value to a large number so that any valid difference found in the array will eventually be smaller than it.
Then, we need two nested loops to iterate over all possible pairs of elements in the array. The outer loop will start from the first index and go until the second-to-last index minus X. The reason for this is that we want to ensure that the selected elements are at least X indices apart.
The inner loop will start from the current index plus X, going until the last index. It iterates over the remaining elements after the current index, ensuring that we're considering pairs that are at least X indices apart. Within this loop, we calculate the absolute difference between the current pair of elements and update the minDiff variable if this calculated difference is smaller.
Here is the pseudocode representation of the algorithm:
1. minDiff = a large number
2. for i = 0 to length of array - X - 1:
3. for j = i + X to length of array - 1:
4. diff = absolute difference between array[i] and array[j]
5. if diff < minDiff:
6. minDiff = diff
7. return minDiff
Using this algorithm, we can efficiently solve the problem of finding the minimum absolute difference between two elements in an array that are at least X indices apart.
In terms of time complexity, the algorithm has nested loops starting from 0 to n, where n is the length of the given array. The inner loop starts from i + X, so the number of iterations gradually decreases as we approach the last element. As a result, the time complexity of this algorithm is approximately O(n^2).
Let's test our algorithm with a few examples. Suppose we have the array [20, 5, 27, 15, 9] and we want to find the minimum absolute difference between two elements that are at least 1 index apart. The algorithm would return 5, as the minimum difference is between 5 and 9.
In another example, let's consider the array [10, 3, 15, 8, 11] and we want to find the minimum absolute difference between two elements that are at least 2 indices apart. The algorithm would return 5, as the minimum difference is between 3 and 8.
In conclusion, finding the minimum absolute difference between two elements in an array becomes more complex when we need to consider a minimum distance or interval between them. However, by applying the algorithm described in this article, we can efficiently solve this problem. By using nested loops and considering all possible pairs of elements, we can find the minimum absolute difference that satisfies the given condition. Understanding this algorithm will undoubtedly enhance your problem-solving skills in the field of computer science and data analysis.