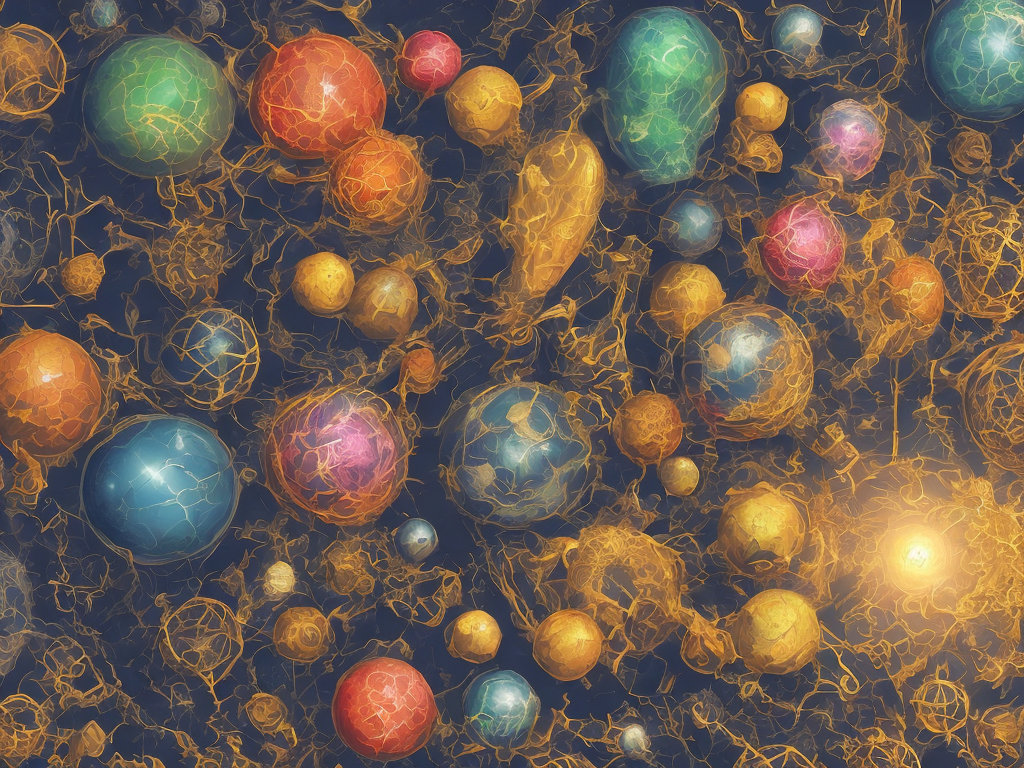
Minimum Absolute Difference Between Elements With Constraint
When working with arrays or lists, it is often necessary to find the minimum absolute difference between two elements. This problem can be solved efficiently using a technique called Two Pointers. Two Pointers is a popular algorithmic approach where two pointers are used to iterate over an array or list simultaneously, usually from both ends or in a specific pattern.
In this article, we will explore the problem of finding the minimum absolute difference between elements in an array, given a constraint. The constraint specifies that the absolute difference must be greater than or equal to a certain value.
Let's consider the following problem statement for a better understanding:
Given an array of integers and an integer value K, find the minimum absolute difference between any two elements in the array such that the absolute difference is greater than or equal to K.
To solve this problem, we can follow these steps:
1. Sort the array in ascending order: Sorting the array helps us identify adjacent elements easily and simplifies the computation of the minimum absolute difference.
2. Initialize two pointers, left and right, to the start and end of the sorted array, respectively.
3. Initialize a variable, minDifference, to store the minimum absolute difference found so far. Set its initial value to a large number.
4. While the right pointer is greater than the left pointer, perform the following steps:
a. Calculate the absolute difference between the elements pointed to by the left and right pointers: absDifference = abs(array[right] - array[left]).
b. If the absolute difference is less than the current minimum difference, update minDifference.
c. If the absolute difference is less than K, move the right pointer one step to the left.
d. Otherwise, move the left pointer one step to the right.
5. After the loop ends, the variable minDifference will contain the minimum absolute difference between any two elements greater than or equal to K.
Let's see an example to understand the algorithm better. Consider the following array: [1, 4, 8, 12, 15] with K = 3.
First, we sort the array, resulting in [1, 4, 8, 12, 15].
Next, we initialize the left pointer to the start of the array (index 0) and the right pointer to the end of the array (index 4).
At the start, the minDifference is set to a large number.
Now, we calculate the absolute difference between array[right] and array[left]. In this case, the difference between 1 and 15 is 14.
Since 14 is greater than the initial minDifference value, we update minDifference to 14.
Next, we compare the absolute difference with K (3). Since 14 is greater, we move the left pointer one step to the right.
Now, the pointers are at indices 1 and 4, respectively.
We calculate the absolute difference between array[right] and array[left], which is 11.
Since 11 is less than the current minDifference (14), we do not update minDifference.
However, 11 is greater than K, so we move the right pointer one step to the left.
The pointers are now at indices 1 and 3.
We calculate the absolute difference between array[right] and array[left], which is 8.
Since 8 is less than the current minDifference (11), we update minDifference to 8.
As 8 is less than K, we move the right pointer one step to the left.
The pointers are now at indices 1 and 2.
Again, we calculate the absolute difference, which is 4.
Since 4 is less than the current minDifference (8), we update minDifference to 4.
This time, 4 is greater than K, so we move the left pointer one step to the right.
The pointers are now at indices 2 and 2.
We calculate the absolute difference between array[right] and array[left], which is 0.
Since 0 is less than the current minDifference (4), we do not update minDifference.
However, 0 is also less than K, so we move the right pointer one step to the left.
The pointers are now at indices 2 and 1.
We calculate the absolute difference, which is 4.
Since 4 is less than the current minDifference (4), we do not update minDifference.
Again, 4 is greater than K, so we move the left pointer one step to the right.
The pointers are now at indices 3 and 1.
We calculate the absolute difference, which is 4.
Since 4 is less than the current minDifference (4), we do not update minDifference.
But this time, 4 is greater than K, so we move the left pointer one step to the right.
The pointers are now at indices 4 and 1.
We calculate the absolute difference, making it 11.
Since 11 is less than the current minDifference (4), we do not update minDifference.
Also, 11 is greater than K, so we move the left pointer one step to the right.
Finally, the while loop ends, and we have found the minimum absolute difference between any two elements greater than or equal to K, which is 4.
The overall time complexity of this algorithm is O(n log n), where n is the number of elements in the array. The sorting operation dominates the time complexity, followed by the linear traversal of the array using two pointers.
In conclusion, the minimum absolute difference between elements can be efficiently found using the Two Pointers technique with a constraint. This approach enables us to solve the problem in an efficient manner by sorting the array and using two pointers to iterate over it. By following the outlined steps, we can compute the minimum absolute difference between elements in an array, taking into account a specified constraint.