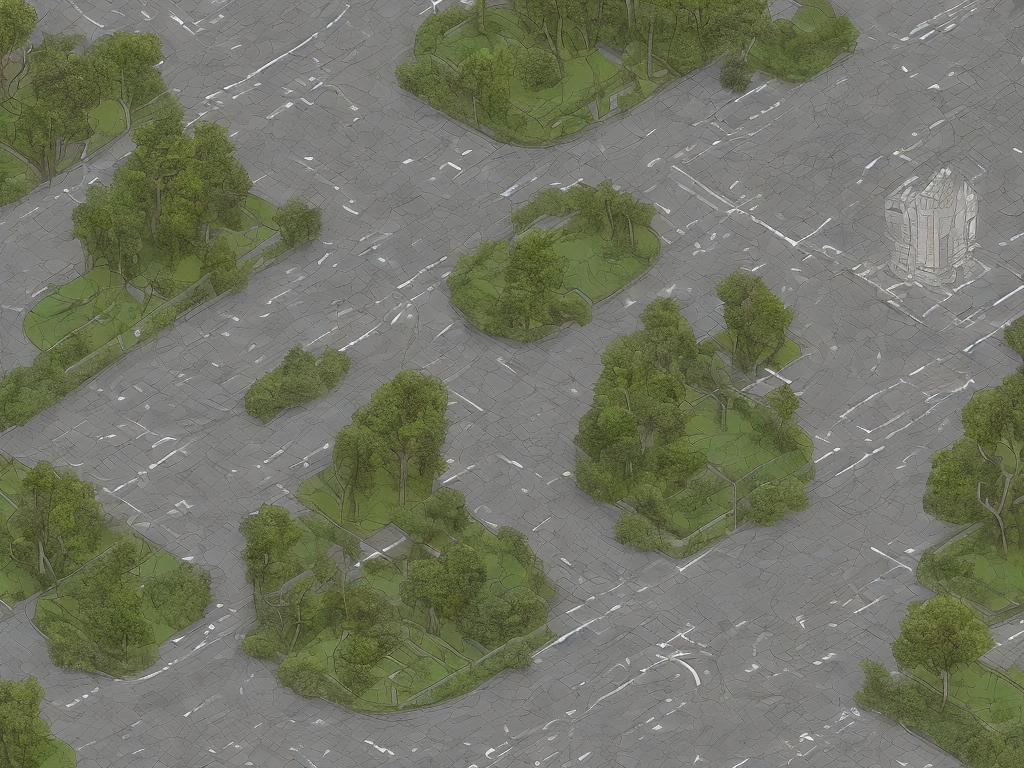
In object-oriented programming (OOP), abstract classes and interfaces are two concepts that can often confuse beginners. Both of them are used in Java, C#, and other high-level programming languages. However, they serve different purposes and have their unique benefits and limitations. In this article, we’ll explore the key differences between abstract classes and interfaces and help you choose the right approach for your programming tasks.
Abstract Classes
An abstract class is a special type of class that can’t be instantiated directly. Instead, it serves as a blue-print or template for derivatives classes, which inherit its properties and methods. Abstract means that the class contains abstract methods, which are declared but not implemented in the abstract class. Abstract methods are meant to be overridden by child classes, which provide their own implementation. The abstract class can also contain non-abstract (concrete) methods that are inherited by child classes without changes.
Here’s an example of an abstract class in Java:
```
public abstract class Shape {
protected int x, y;
public void moveTo(int newX, int newY) {
System.out.println("Move shape to (" + newX + ", " + newY + ")");
x = newX;
y = newY;
}
public abstract double area(); // declare abstract method
}
```
In this example, we create an abstract class called Shape, which has two instance variables x and y and two methods – moveTo and area. The moveTo method is a concrete method and has a default implementation that can be used by child classes. The area method, on the other hand, is an abstract method and has no implementation in the Shape class. Child classes of Shape must implement their versions of the area method.
Here’s an example of how a child class can inherit from the Shape class:
```
public class Rectangle extends Shape {
private int width, height;
public Rectangle(int x, int y, int width, int height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
@Override
public double area() {
return width * height;
}
}
```
In this example, we create a child class of Shape called Rectangle. The Rectangle class has its own instance variables width and height, and a constructor that initializes them along with the x and y inherited from the parent Shape class. The Rectangle class also overrides the area method and provides its own implementation (the area of a rectangle is width times height).
So, what are the benefits of using abstract classes?
- Abstract classes provide a base template for child classes, which can save time and effort in creating similar classes.
- Abstract classes can contain both abstract and concrete methods, giving child classes flexibility in implementing only the methods they need to change.
- Abstract classes can also have instance variables, constructors, and non-public methods, which are not possible in interfaces.
Interfaces
Interfaces, like abstract classes, are also templates for classes. However, interfaces serve a different purpose than abstract classes. An interface is a collection of abstract methods, without any implementation. An interface cannot be instantiated, and its methods must be implemented by the class that uses the interface. A class that implements an interface must provide its own implementation of all the interface’s methods.
Here’s an example of an interface in Java:
```
public interface Drawable {
void draw();
void resize(double factor);
String getDescription();
}
```
In this example, we create an interface called Drawable, which has three abstract methods – draw, resize, and getDescription. The interface does not provide any implementation for these methods. Any class that implements the Drawable interface must implement all these methods according to their functionality.
Here’s an example of how a class implements the Drawable interface:
```
public class Circle implements Drawable {
private double radius;
private String description;
public Circle(double radius, String description) {
this.radius = radius;
this.description = description;
}
@Override
public void draw() {
System.out.println("Drawing " + description + " circle");
// code to draw circle on screen
}
@Override
public void resize(double factor) {
radius *= factor;
}
@Override
public String getDescription() {
return description;
}
}
```
In this example, we create a class called Circle that implements the Drawable interface. The Circle class has its own instance variables called radius and description, and a constructor that initializes them. The Circle class also implements all the abstract methods of the Drawable interface, providing its own implementation for each method.
So, what are the benefits of using interfaces?
- Interfaces provide a way to enforce a standard functionality to all classes that use the interface.
- Interfaces can be implemented by any class, regardless of its inheritance hierarchy. This allows us to create classes that have certain functionality without restricting them to a specific base class.
- Interfaces can be used to achieve polymorphism, which allows us to write code that works with any object that implements the interface, regardless of its type.
Abstract Classes vs. Interfaces
Based on the examples given above, we can sum up the main differences between abstract classes and interfaces:
- Abstract classes can have both abstract and concrete methods. Interfaces only have abstract methods.
- A class can only inherit from one abstract class, but it can implement multiple interfaces.
- Abstract classes can have instance variables, constructors, and non-public methods. Interfaces cannot have any of these.
- Abstract classes provide a partial implementation of a class, while interfaces provide a standard functionality which must be implemented in full by any class that uses it.
So, which one should you use, abstract classes or interfaces?
The answer is, it depends on your specific programming task and requirements. Generally, you should use an abstract class when you want to create a base class for a group of similar classes, and you want to provide some implementation that can be inherited by those classes. You should use an interface when you want to enforce a standard behavior for a group of classes, and you want to allow those classes to have their own implementation of that behavior.
In conclusion, abstract classes and interfaces are both critical tools in OOP, and they have different applications and benefits. Understanding the differences between them will help you choose the right approach for your programming tasks, and write better, cleaner, and more efficient code.