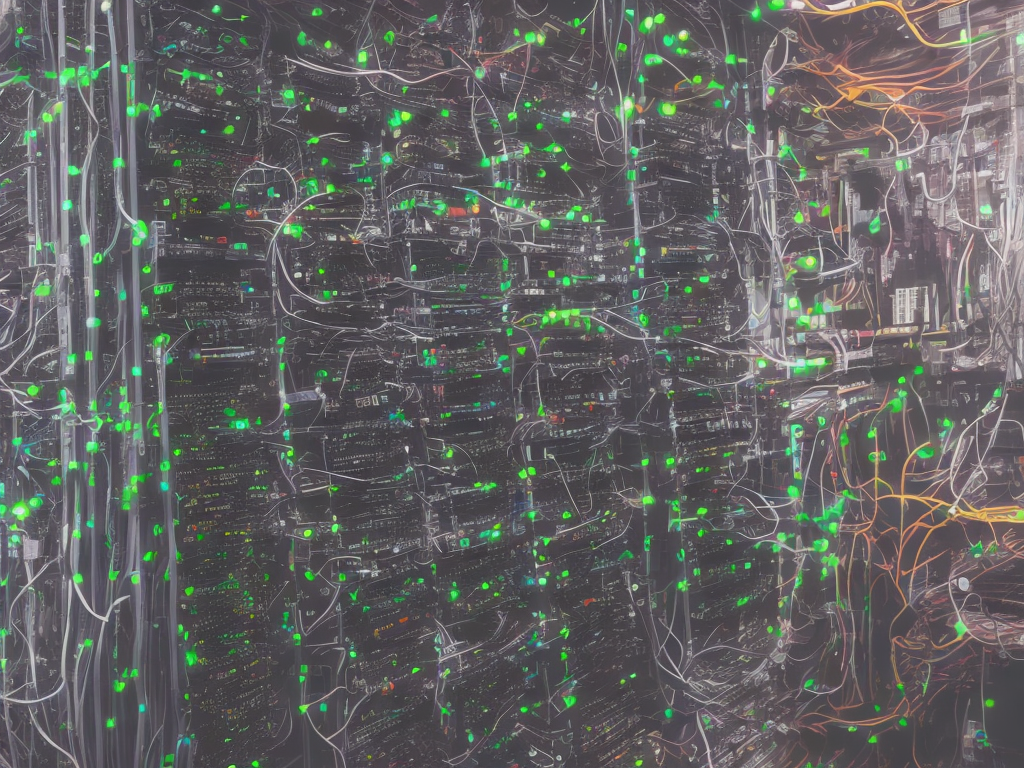
When it comes to developing web applications in Java, it's important to understand the difference between GenericServlet and HttpServlet. Both of these classes are important for creating web applications and are widely used by developers worldwide. Knowing when to use each one can greatly improve your application's overall performance.
GenericServlet
GenericServlet is a class in Java's servlet API that provides an abstract class for building servlets. This class is the base class for all servlet classes in the Java Servlet API and provides a set of methods that can be overridden by sub-classes to implement specific functionality. The GenericServlet class provides support for types of requests that are not specific to HTTP.
This means that any class that extends the GenericServlet class can process any type of request. For instance, you can use this class to handle requests for other types of protocols such as FTP, Gopher, SMTP, etc. To do this, you would just need to override the service() method to handle each request type.
The GenericServlet has four main methods that are commonly used when creating servlets:
1. init(ServletConfig config) – This method gets called when the servlet is first loaded or initialized.
2. service(ServletRequest req, ServletResponse res) – This method gets called every time a request comes in. This is where most of the work happens in a servlet.
3. getServletConfig() – This method returns a ServletConfig object which provides information about the servlet instance.
4. destroy() – This method gets called when the servlet is being unloaded or shutdown. It's used to clean up resources or data held by the servlet.
HttpServlet
HttpServlet is a specific implementation of the GenericServlet class that's optimized for handling HTTP requests and responses. This class is used for building web applications that work with the HTTP protocol. The HttpServlet class provides an easy-to-use interface for creating web applications in Java. Developers can use this class to create web pages, handle HTTP requests, and manipulate cookies and sessions.
Unlike the GenericServlet, HttpServlet is designed for HTTP-specific functionality. Its service() method takes a request object that implements the HttpServletRequest interface and a response object that implements the HttpServletResponse interface. This makes it easy to work with these objects while coding web apps.
The HttpServlet class has a few more methods that are specific to HTTP:
1. doGet(HttpServletRequest request, HttpServletResponse response) – This method is used to handle GET requests.
2. doPost(HttpServletRequest request, HttpServletResponse response) – This method is used to handle POST requests.
3. doPut(HttpServletRequest request, HttpServletResponse response) – This method is used to handle PUT requests.
4. doDelete(HttpServletRequest request, HttpServletResponse response) – This method is used to handle DELETE requests.
5. init() – This method is called by the container to initialize the servlet. It's used to perform any initializations that are required before the servlet can handle requests.
6. destroy() – This method is called by the container to destroy the servlet. It's used to release any resources held by the servlet.
The HttpServlet class also provides a number of convenience methods that make it easy to get request and response data such as headers, cookies, parameters, and so on. These methods allow developers to work with the HTTP data more easily and abstract away some of the lower-level details.
Key Differences
The main difference between GenericServlet and HttpServlet lies in the fact that HttpServlet is designed specifically for web applications that work with the HTTP protocol, while GenericServlet is designed to handle more than just HTTP requests. This means that when you're building a web application, using the HttpServlet class is generally the better choice, as it provides specific functionality for HTTP requests and responses.
Another key difference between the two classes is that GenericServlet is an abstract class, while HttpServlet is a concrete class. This means that GenericServlet cannot be instantiated directly, but has to be subclassed. HttpServlet, on the other hand, can be instantiated directly and used immediately.
Finally, HttpServlet has a couple of additional methods that are specific to HTTP requests, such as doGet(), doPost(), etc. These methods aren't provided by GenericServlet and are required when handling HTTP requests.
Conclusion
In summary, both GenericServlet and HttpServlet are important classes for building web applications in Java. While GenericServlet provides an abstract class for building servlets that can handle any type of request, HttpServlet is specifically designed for handling HTTP requests and provides specific functionality for working with these requests. When building a web application, it's generally a good idea to use the HttpServlet class over GenericServlet, as it provides better support for HTTP requests and is easier to work with.