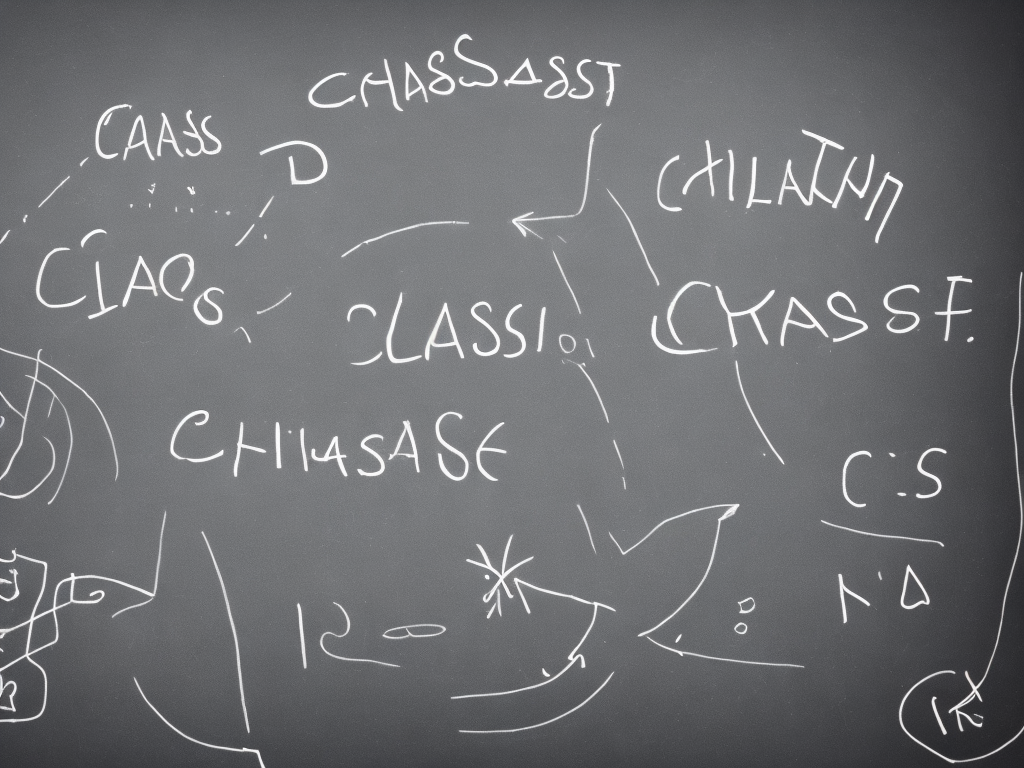
React is an open-source user interface library maintained by Facebook. It is used to create efficient and reusable UI components. These components can be either functional or class-based.
React components serve as building blocks for constructing a user interface. Each component is designed to handle a specific task within the application. Components can be reused throughout the app, making the development process faster and less complex.
React components can be created using two different approaches - class components and functional components. While both of these approaches serve the same purpose, they differ in how they are created, used, and their intended purpose.
In this article, we will explore the difference between class components and functional components in React.
Functional Components
Functional components, also known as stateless components, are pure JavaScript functions that take in properties (props) and return a React element. The props object contains data that is passed down to the component from its parent component.
Functional components are easier to read, test, and optimize than class components because they don't have any state or lifecycle methods. They are also simpler to understand and use, making them an ideal choice for small applications, or when creating reusable UI elements.
Functional components are typically used for rendering UI elements, such as buttons, forms, and other simple displays. They are also used to create higher-order components (HOC), which are essentially components that wrap around other components to provide additional functionality.
Functional components can be created using the new ES6 arrow functions, as shown below:
```
const Greeting = (props) => {
return
Hello, {props.name}!
;}
```
Class Components
Class components, also known as stateful components, are JavaScript classes that extend the React Component class. They have state and lifecycle methods that can be used to manage data and handle the rendering of the component.
Unlike functional components, class components can create and manage their own state object. The state object can be updated using the setState() method, which triggers a re-render of the component when the state changes.
Class components have access to several lifecycle methods, which are methods that are called at specific times during the life of a component. These methods allow developers to perform certain actions, such as data fetching or UI updating, when certain events occur.
Class components are typically used for more complex functionality, such as handling user input, managing data, and making API calls. They can also be used to create reusable UI elements, although this may require more code than a functional component.
Class components can be created using the class keyword, as shown below:
```
class Greeting extends React.Component {
constructor(props) {
super(props);
this.state = { name: props.name };
}
render() {
return
Hello, {this.state.name}!
;}
}
```
The Difference Between Functional and Class Components
Both functional and class components are used to create React UI elements, but they differ in several key ways.
One of the biggest differences between functional and class components is that functional components are simpler and easier to read than class components. They are also easier to test and optimize because they don't have any state or lifecycle methods.
On the other hand, class components are more powerful and flexible than functional components because they have state and lifecycle methods. This makes them better suited for handling complex data, making API calls, and managing user input.
Functional components are typically used for simple UI elements, such as buttons and forms, or when creating reusable UI elements. Class components are used for more complex functionality, such as managing data and handling user input.
Another significant difference between functional and class components is the way they handle state. Functional components can't have state objects, and they can't use setState() to update data. Instead, they rely on props to receive data from the parent component.
Class components, on the other hand, have access to the this.state object, which can be used to store and update data. They can also use setState() to update the state object and trigger a re-render of the component.
Conclusions
In conclusion, functional components and class components are two different approaches to creating React UI elements. Functional components are simpler and more lightweight, while class components are more powerful and flexible.
Functional components work best for simple UI elements or when creating reusable components. Class components are better suited for handling complex data, making API calls, and managing user input.
Ultimately, the choice of which approach to use depends on the specific needs of the application. In some cases, a functional component may be sufficient, while in others, a class component may be required to handle more complex functionality.