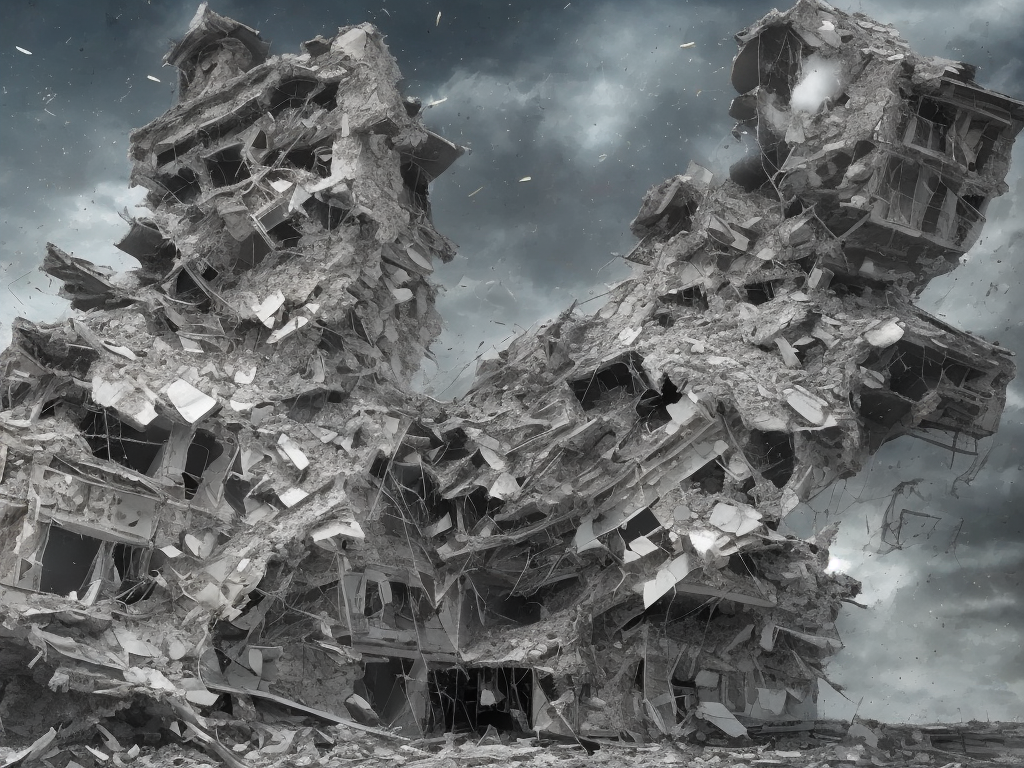
When it comes to programming, implode and explode are two commonly used functions that are crucial in working with arrays and strings. Both are used to manipulate data within a program, but they have different functions and can achieve different outcomes. In this article, we will explore the difference between implode and explode, how they work, and when to use them.
Explode Explained
Explode is a PHP function that breaks down strings into an array of substrings, based on a designated separator. The function takes two parameters: the delimiter and the string. The delimiter is used to separate the string into substrings, and the result of the function is an array of those substrings.
Consider the following example:
```
$string = "Lorem ipsum dolor sit amet.";
$delimiter = " ";
$array = explode($delimiter, $string);
```
In this example, we have assigned a string to the variable `$string` and a delimiter to `$delimiter`. Since we have chosen a space as our delimiter, the explode function will break down the string into substrings based on every space. The resulting array will look like this:
```
Array
(
[0] => Lorem
[1] => ipsum
[2] => dolor
[3] => sit
[4] => amet.
)
```
As you can see, each word in the string has been separated into its own element in the array. Explode is particularly useful when you want to extract certain information from a larger string, such as breaking down an email address into its username and domain.
Implode Explained
Implode, on the other hand, is a function used to join the elements of an array together into a single string. The function takes two parameters: the glue and the array. The glue is used to concatenate the elements of the array, and the result is a string.
Consider the following example:
```
$array = array('apple', 'banana', 'orange');
$glue = ", ";
$string = implode($glue, $array);
```
In this example, we have assigned an array of fruits to the variable `$array` and a glue to `$glue`. The glue in this example is a comma followed by a space. The implode function will join the elements of the array together using the glue, resulting in the following string:
```
"apple, banana, orange"
```
Implode is particularly useful when you want to join elements of an array together to form a string. For instance, if you want to display a list of products separated by commas, you could use implode to join the product names together with commas.
It is important to note that both implode and explode are specific to PHP, and other programming languages may have different functions with similar functions.
The Difference
At their core, implode and explode are both used to manipulate arrays and strings, but they are fundamentally different in how they manipulate data. Explode is used to break down a string into an array of substrings, while implode is used to join elements of an array into a single string.
Another key difference between the two functions is the order of the parameters. With explode, the delimiter parameter comes before the string, but with implode, the glue parameter comes before the array. This is important to keep in mind when calling the functions and can lead to errors if the parameters are accidentally flipped.
When to Use Explode and Implode
When to use explode and implode depends entirely on the situation. If you have a string that needs to be divided into smaller pieces, explode is the function to use. Similarly, if you have an array of data that needs to be combined into a single string, use implode.
Explode is often used in parsing data from files or websites where there is a specific pattern in the data that can be used as a delimiter. For example, if you were reading a comma-separated values (CSV) file, you could use explode to separate each line of the file into an array of values.
Implode is often used in creating visually appealing output for users. For instance, if you have an array containing all of the elements of a list, you could use implode to join them together into a single string with commas between each element. This could be used to display a list of products, services or other elements to a user.
Conclusion
In conclusion, both implode and explode are crucial functions used in PHP programming. While both functions manipulate data within arrays and strings, they have different functions and can achieve different outcomes. Explode is used to break down a string into substrings, while implode is used to join elements of an array into a single string. By understanding the differences between the two functions and when to use them, you can improve your programming efficiency and achieve better results.